Use the SDK
The server SDK for Java supports various features. The following sample code provides an example on how to query the snapshot configurations of a streaming domain:
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.live.model.v20161101.DescribeLiveSnapshotConfigRequest;
import com.aliyuncs.live.model.v20161101.DescribeLiveSnapshotConfigResponse;
import com.aliyuncs.profile.DefaultProfile;
public class SdkUseDemo {
public static void main(String[] args) {
DefaultProfile profile = DefaultProfile.getProfile("<regionId>", "<ALIBABA_CLOUD_ACCESS_KEY_ID>", "<ALIBABA_CLOUD_ACCESS_KEY_SECRET>");
IAcsClient client = new DefaultAcsClient(profile);
DescribeLiveSnapshotConfigRequest describeLiveStreamSnapshotInfoRequest=new DescribeLiveSnapshotConfigRequest();
describeLiveStreamSnapshotInfoRequest.setDomainName("<DomainName>");
DescribeLiveSnapshotConfigResponse describeLiveSnapshotConfigResponse = null;
try {
describeLiveSnapshotConfigResponse = client.getAcsResponse(describeLiveStreamSnapshotInfoRequest);
} catch (ClientException e) {
e.printStackTrace();
}
}
}
Note
You can use the sample code to test the SDK functionality. Replace the placeholders with actual values in the sample code.
The regionId parameter specifies the ID of the service region. To obtain the region ID, see Endpoints.
The ALIBABA_CLOUD_ACCESS_KEY_ID and ALIBABA_CLOUD_ACCESS_KEY_SECRET parameters specify the AccessKey pair. For information about how to obtain an AccessKey pair, see Obtain an AccessKey pair.
DescribeLiveSnapshotConfigRequest and DescribeLiveSnapshotConfigResponse are request and response classes for querying snapshot configurations.
For information about request and response parameters, see DescribeLiveSnapshotConfig.
The APIs provided by ApsaraVideo Live have limits on the maximum number of queries per second (QPS). For information about QPS limits, see Flow control information.
In this example, DescribeLiveSnapshotConfigResponse is a deserialized response object. If you want to work directly with the raw HTTP response, change the client.getAcsResponse method to the client.doAction method. Sample code:
HttpResponse httpResponse=client.doAction(describeLiveStreamSnapshotInfoRequest);
int status=httpResponse.getStatus();
Note:
HTTP status codes from 200 to 299 indicate successful calls.
HTTP status codes from 300 to 499 trigger the SDK to throw a ClientException, which indicates a client error.
HTTP status codes 500 and above trigger the SDK to throw a ServerException, which indicates a server error.
We recommend that you save the AccessKey pair in a properties file so that the SDK can get the AccessKey pair when needed. The following sample code shows how to configure it in a Spring Boot-based project:
# The AccessKey ID and AccessKey secret requested by the server SDK.
live.accessKeyId=<yourAccessKeyId>
live.accessKeySecret=<yourAccessKeySecret>
@Value("${live.accessKeyId}")
private String accessKeyId;
@Value("${live.accessKeySecret}")
private String accessKeySecret;
Important
When you use ApsaraVideo Live SDKs, adhere to the following naming conventions:
The following table lists some common APIs of ApsaraVideo Live.
API | Request class | Response class |
Access control
In addition to system policies that authorize RAM users to access APIs, you can create custom policies to implement finer-grained access control based on the resource type. For example, you can configure a policy to grant RAM User A access to an API operation only on Domain A and RAM User B access only on Domain B. For information about how to implement access control, see Create a custom policy.
The API reference provides the authorization information of each API, describing what permissions you can grant to a RAM user or role for using the operation. The following figure shows the authorization information of the AddLiveAppSnapshotConfig operation.
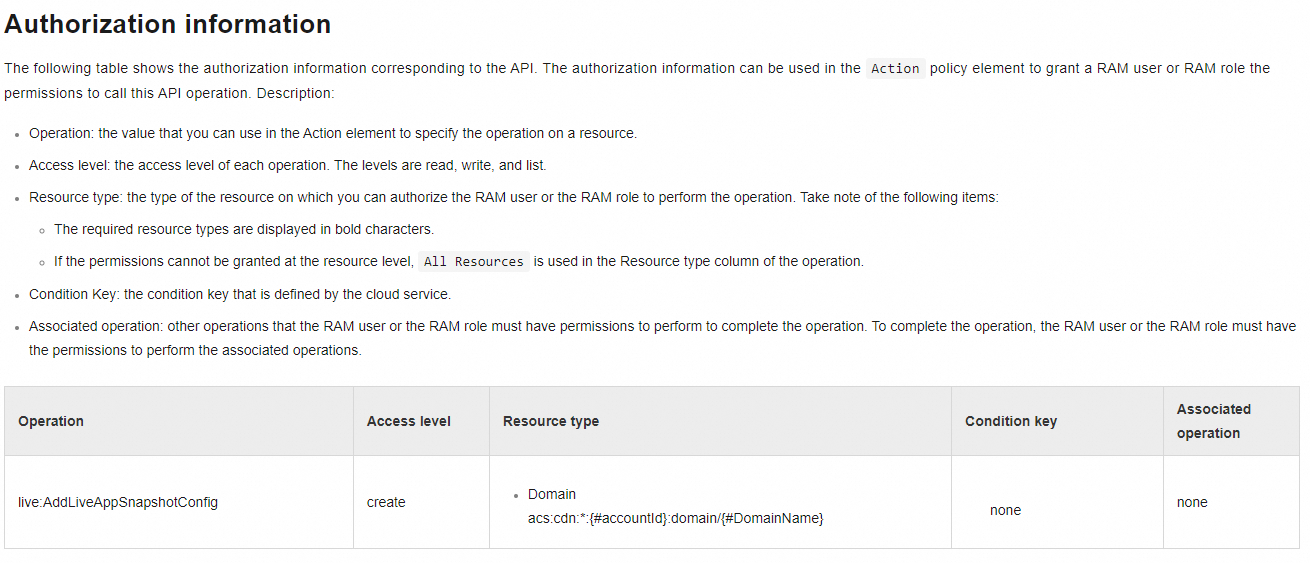
Note
For information about access control supported by each API, see RAM authorization.