This topic describes how to call the API operations for managing Alibaba Cloud tickets by using a RAM identity. To do this, you can first create a RAM user or a RAM role, and authorize the RAM user or RAM role to call the related API operations. Then, you can programmatically interact with the Alibaba Cloud ticket service. This topic also provides some sample code that you can use to call the relevant API operations by using RAM identities.
Create a RAM identity
Create a RAM user
Log on to the Alibaba Cloud RAM console (https://ram.console.aliyun.com/users), and choose Identities > Users in the left-side navigation pane. On the page that appears, click Create User. Make sure that you select OpenAPI Access, as shown in the following figure.
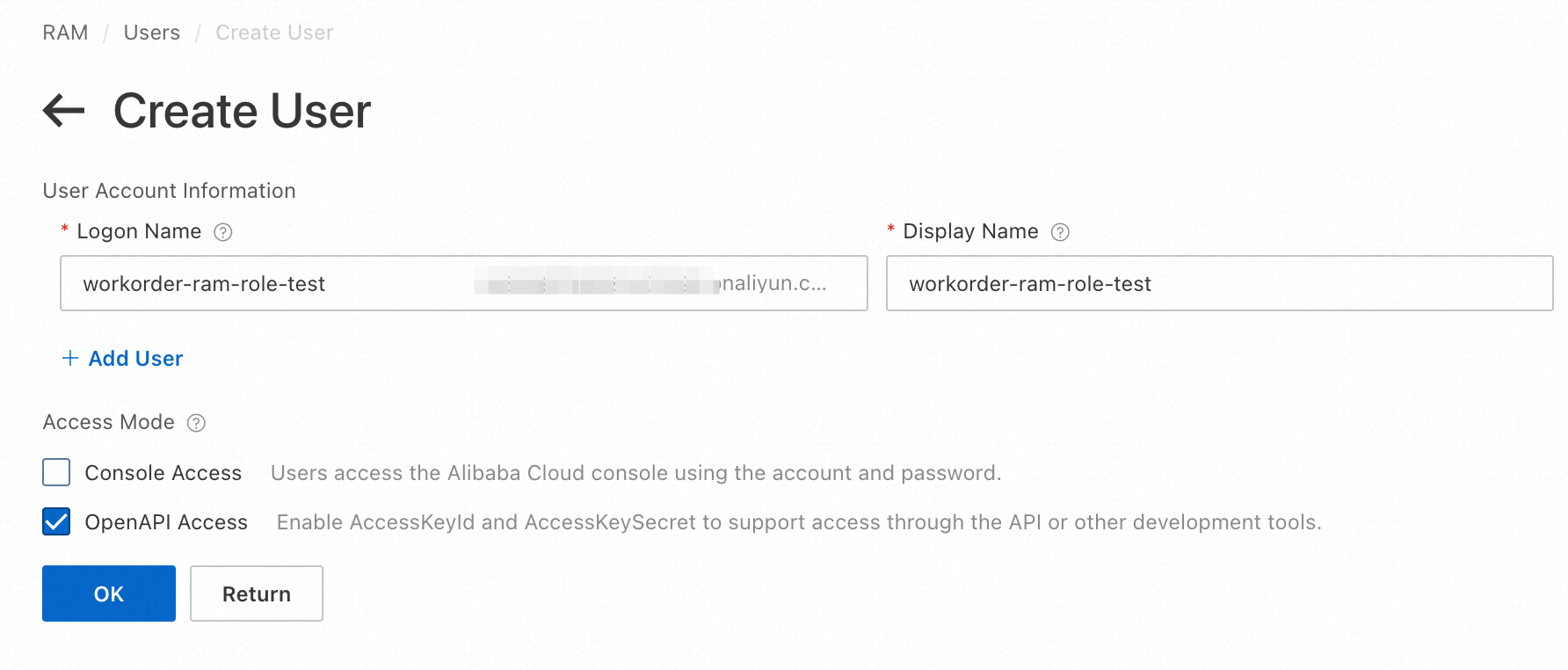
Create a RAM role
Log on to the Alibaba Cloud RAM console (https://ram.console.aliyun.com/roles) and choose Identities > Roles in the left-side navigation pane. On the page that appears, click Create Role.
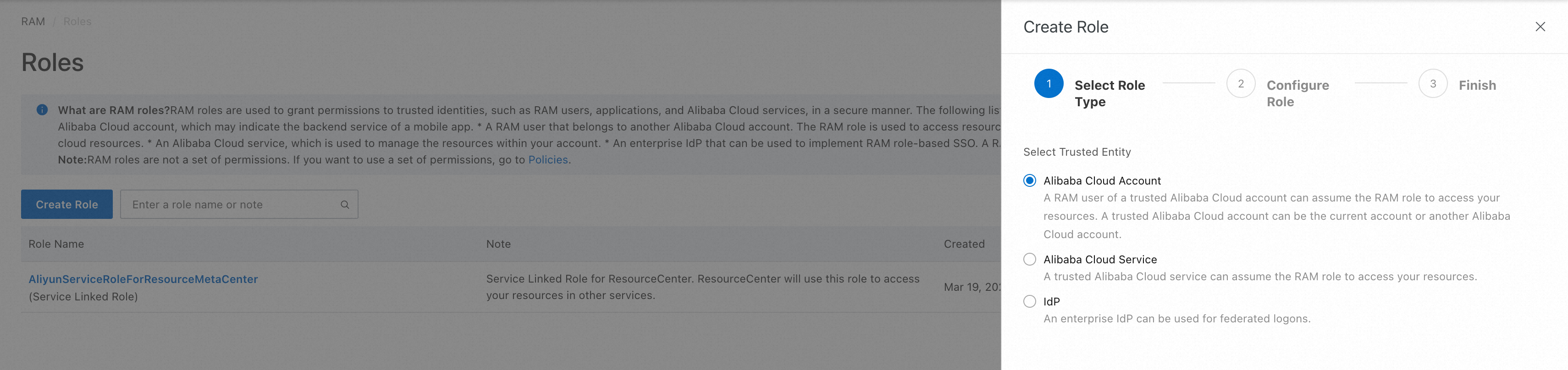
Specify the name of the RAM role that you want to create. In this example, the RAM role is named workorder-ram-role-test, as shown in the following figure.
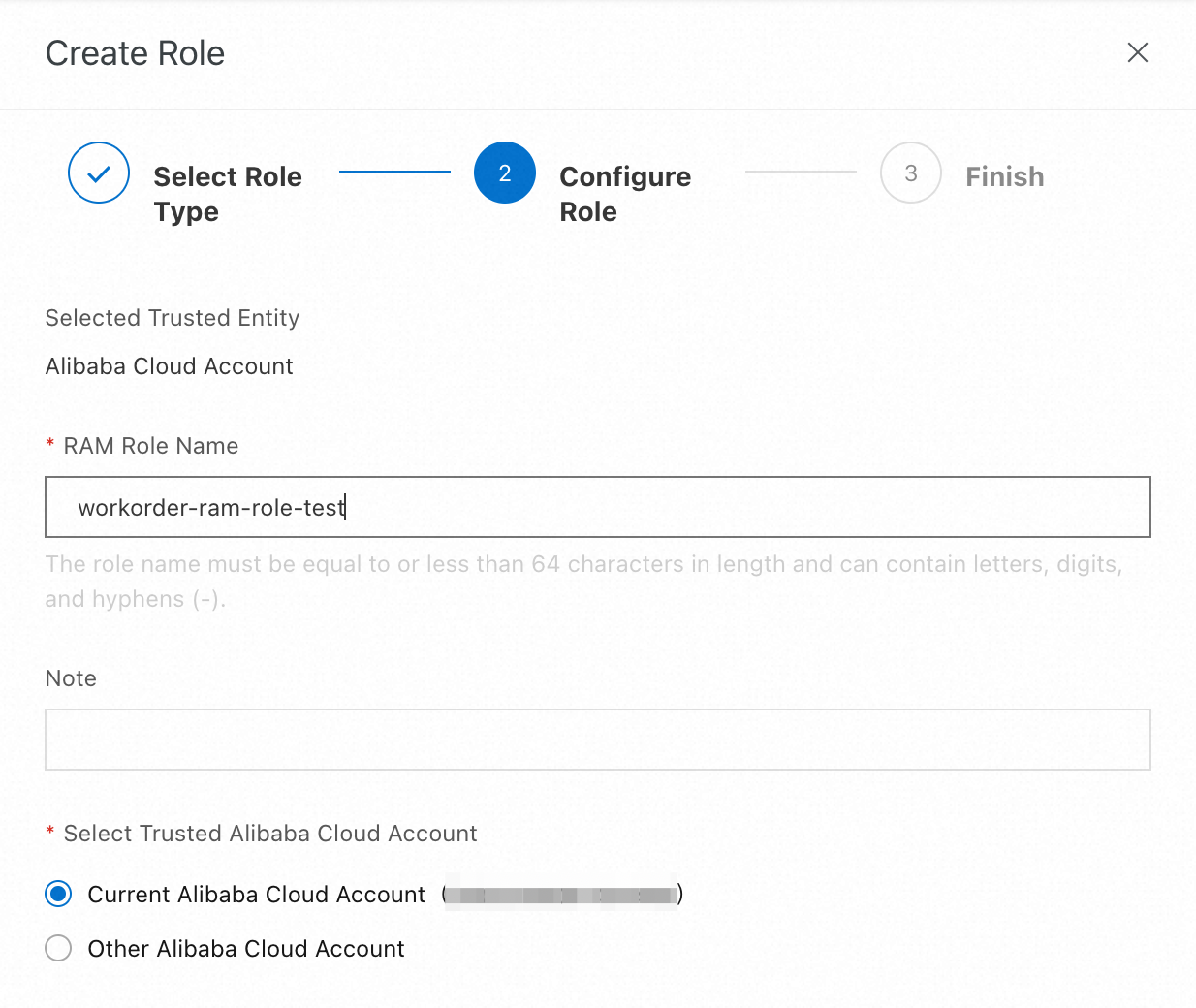
Authorize the RAM identity to access the Alibaba Cloud ticket service
Authorize a RAM user to access the Alibaba Cloud ticket service
In the Alibaba Cloud RAM console, choose Identities > Users in the left-side navigation pane, find the RAM user you just created, and click Add Permissions in the Actions column. In the Add Permissions panel, select the AliyunSupportFullAccess policy for System Policy. You can enter AliyunSupportFullAccess in the search box to find the policy. Make sure that the AliyunSupportFullAccess policy is listed in the Selected column on the right and click OK. Then, the RAM user gains access to the Alibaba Cloud ticket service.
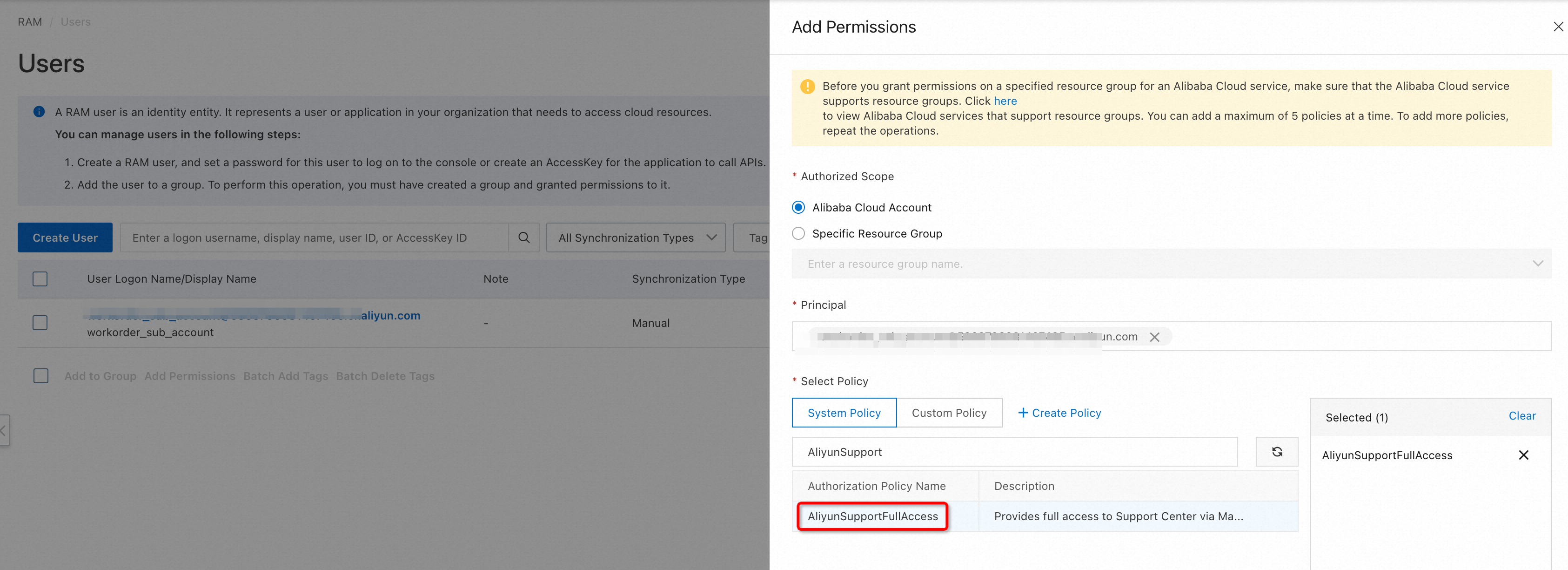
Authorize a RAM role to access the Alibaba Cloud ticket service
In the Alibaba Cloud RAM console, choose Identities > Roles in the left-side navigation pane, find the RAM role that you just created, and click Add Permissions in the Actions column. In the Add Permissions panel, select the AliyunSupportFullAccess policy for System Policy. You can enter AliyunSupportFullAccess in the search box to find the policy. Make sure that the AliyunSupportFullAccess policy is listed in the Selected column on the right and click OK. Then, the RAM role gains access to the Alibaba Cloud ticket service.
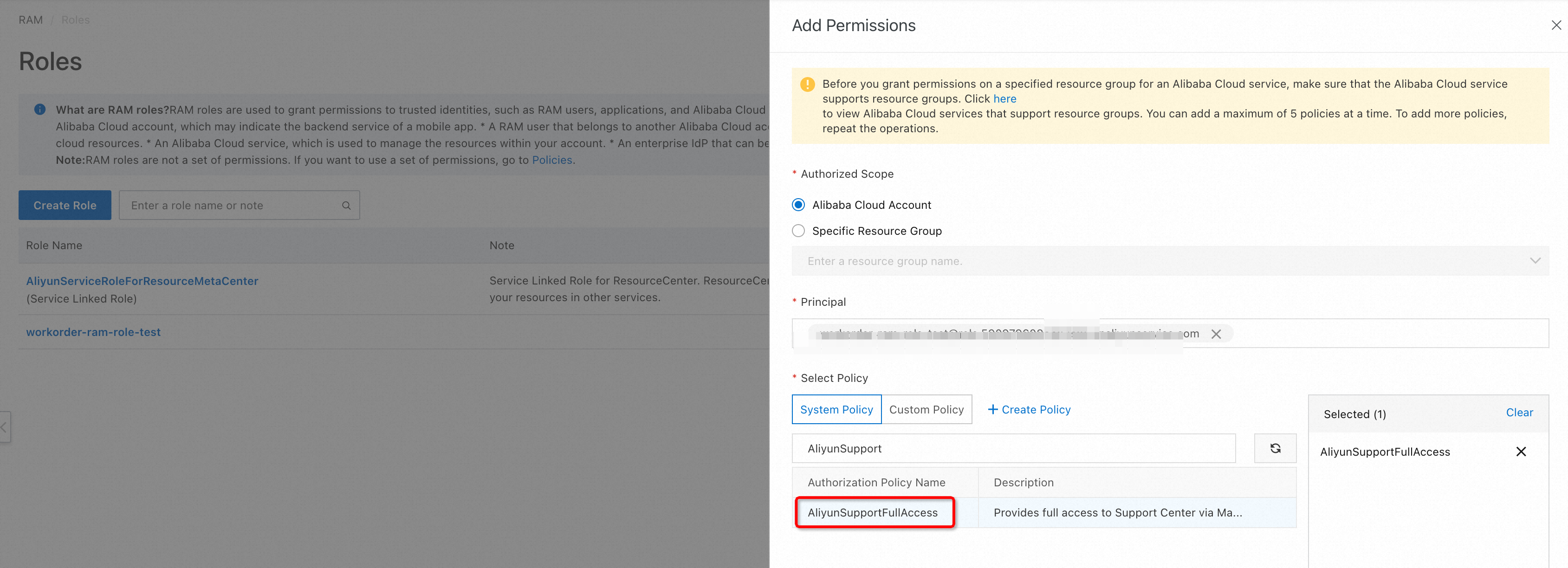
Usage notes for authorizing a RAM identity the permissions to the ticket system
1. As described in the previous instructions, you can attach the system policy
AliyunSupportFullAccess to a RAM User or a RAM Role to grant it full permissions to the ticket system, including the permissions required to create tickets, reply to tickets, and view tickets.
2. Some users manage permissions by configuring permission policy expressions. When the ticket system authenticates a user request, both support and workorder parameters are verified. If either
verification result is ExplicitDeny, the response to the user request is Deny. Therefore, if you use the Deny effect and the StringNotLike parameter to allow specified actions, you must add support:* and workorder:* to the action list of the StringNotLike parameter. See the following sample code:
{
"Action": "*",
"Condition": {
"StringNotLike": {
"Action": [
"*:BatchGet*",
"*:BatchQuery*",
"*:Describe*",
"*:Get*",
"*:List*",
"*:Query*",
"sts:*",
"support:*",
"workorder:*"
]
}
},
"Effect": "Deny",
"Resource": "*"
}
3. You can select one of the previously described authorization methods. The method that uses the system policy is recommended.
Call Alibaba Cloud ticket service API operations by using a RAM identity
Dependency configuration
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-core</artifactId>
<version>4.6.1</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>aliyun-java-sdk-workorder</artifactId>
<version>3.0.0</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>credentials-java</artifactId>
<version>0.2.11</version>
</dependency>
Call the API operations by using a RAM user
import com.google.gson.Gson;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.exceptions.ServerException;
import com.aliyuncs.profile.DefaultProfile;
import com.aliyuncs.workorder.model.v20200326.*;
import com.aliyuncs.workorder.model.v20200326.*;
public class Test {
public static void main(String[] args) throws Exception {
new Test().callWorkorderAPIByRamAKAndSK();
}
public void callWorkorderAPIByRamAKAndSK() {
DefaultProfile profile = DefaultProfile.getProfile("ap-southeast-1", System.getenv("ALIBABA_CLOUD_ACCESS_KEY_ID"), System.getenv("ALIBABA_CLOUD_ACCESS_KEY_SECRET"));
IAcsClient acsClient = new DefaultAcsClient(profile);
ListCategoriesRequest request = new ListCategoriesRequest();
request.setLanguage("en");
request.setProductCode("ecs");
try {
ListCategoriesResponse response = acsClient.getAcsResponse(request);
System.out.println(new Gson().toJson(response));
} catch (ServerException e) {
e.printStackTrace();
} catch (ClientException e) {
System.out.println("ErrCode:" + e.getErrCode());
System.out.println("ErrMsg:" + e.getErrMsg());
System.out.println("RequestId:" + e.getRequestId());
}
}
}
Call the API operations by using a RAM role
For more information about how to configure credentials and an STS token, see https://www.alibabacloud.com/help/en/alibaba-cloud-sdk-262060/latest/credentials-settings-2#p-x9q-h7t-bpx.
import com.google.gson.Gson;
import com.aliyun.credentials.Client;
import com.aliyun.credentials.models.Config;
import com.aliyuncs.DefaultAcsClient;
import com.aliyuncs.IAcsClient;
import com.aliyuncs.exceptions.ClientException;
import com.aliyuncs.exceptions.ServerException;
import com.aliyuncs.profile.DefaultProfile;
import com.aliyuncs.workorder.model.v20200326.*;
import com.aliyuncs.workorder.model.v20200326.*;
public class Test {
public static void main(String[] args) throws Exception {
new Test().callWorkorderAPIByRamRoleSts();
}
public void callWorkorderAPIByRamRoleSts() {
Config config = new Config();
config.type = "ram_role_arn";
config.accessKeyId = System.getenv("ALIBABA_CLOUD_ACCESS_KEY_ID");
config.accessKeySecret = System.getenv("ALIBABA_CLOUD_ACCESS_KEY_SECRET");
config.roleArn = "acs:ram::5135629276002605:role/support-role";
config.roleSessionName = "test_support_role_session_name";
config.roleSessionExpiration = 3600;
Client client = new Client(config);
DefaultProfile profile = DefaultProfile.getProfile(
"ap-southeast-1",
System.getenv("ALIBABA_CLOUD_ACCESS_KEY_ID"),
System.getenv("ALIBABA_CLOUD_ACCESS_KEY_SECRET"),
System.getenv("ALIBABA_CLOUD_SECURITY_TOKEN"));
IAcsClient acsClient = new DefaultAcsClient(profile);
ListCategoriesRequest request = new ListCategoriesRequest();
request.setLanguage("en");
request.setProductCode("ecs");
try {
ListCategoriesResponse response = acsClient.getAcsResponse(request);
System.out.println(new Gson().toJson(response));
} catch (ServerException e) {
e.printStackTrace();
} catch (ClientException e) {
System.out.println("ErrCode:" + e.getErrCode());
System.out.println("ErrMsg:" + e.getErrMsg());
System.out.println("RequestId:" + e.getRequestId());
}
}
}
To obtain the value of config.roleArn, go to the Alibaba Cloud RAM console. Choose Identities > Roles in the left-side navigation pane and click the role that you want to use. You can find the ARN of the role on the details page that appears, as shown in the following figure.
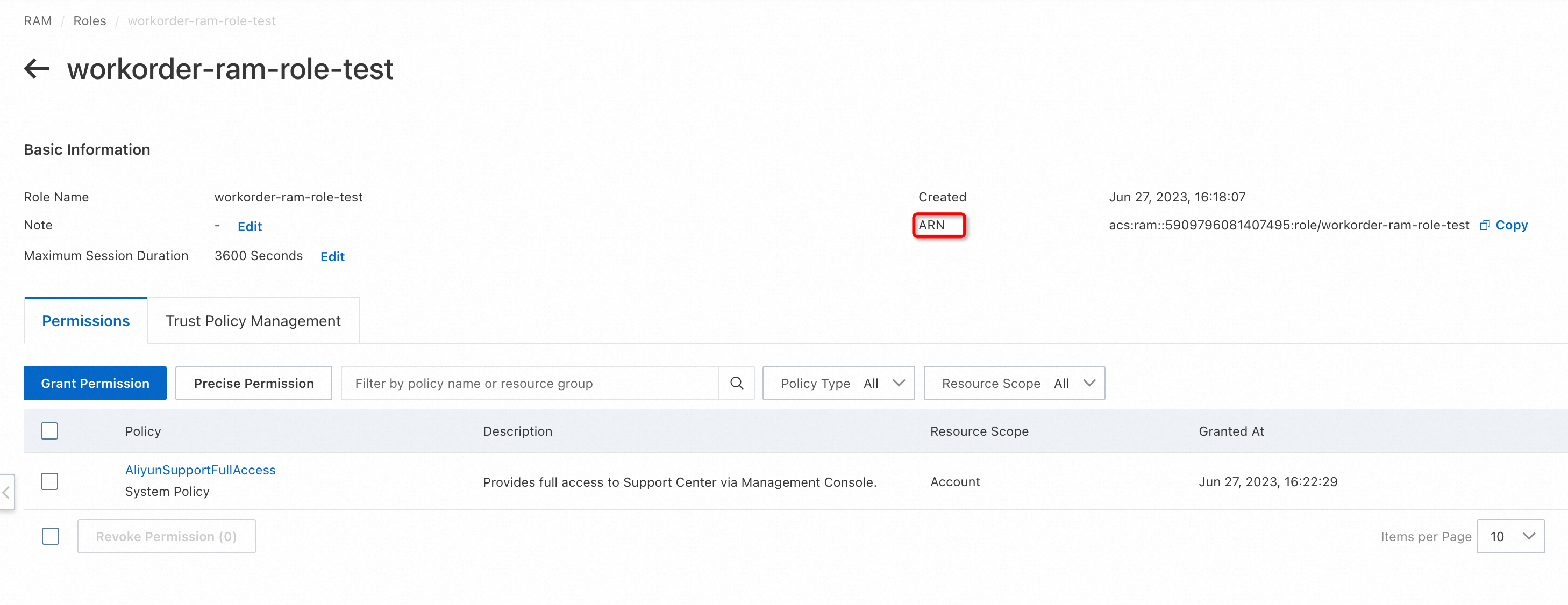
We recommend that you use a descriptive role session name as the value for config.roleSessionName.