AUTitleBar provides a title bar with a Back button, title text, a progress bar, a left button (text and icon), and a right button (text and icon).
Sample image
The AUTitleBar control with white background is provided by default.
Dependency
See Quick start.
API description
/**
* Set the button drawable.
* @param iconView
* @param resId
*/
public void setBtnImage(AUIconView iconView, int resId);
/**
* Set the button size and color.
* @param iconView
* @param size
* @param color
*/
public void setIconFont(AUIconView iconView, int size, int color);
/**
* Get the Back button.
* @return
*/
public AUIconView getBackButton() ;
/**
* Get the left button.
* @return
*/
public AURelativeLayout getLeftButton() ;
/**
* Get the right button.
* @return
*/
public AURelativeLayout getRightButton() ;
/**
* Get the loading icon.
* @return
*/
public AUProgressBar getProgressBar() ;
/**
* Get the title text view.
* @return
*/
public AUTextView getTitleText() ;
/**
* Get the title container.
* @return
*/
public AURelativeLayout getTitleContainer() ;
/**
* Get the title bar area.
* @return
*/
public AURelativeLayout getTitleBarRelative() ;
@Override
public void setBackgroundDrawable(Drawable backgroundDrawable) ;
/**
* Set the progress circular.
* @param progressDrawable
*/
public void setProgressBarDrawable(Drawable progressDrawable) ;
/**
* Set the text and style of the title. If you need to use the default text and style, set text, textsize, and textcolor to null or 0.
* @param text
* @param textSize
* @param textColor
*/
public void setTitleText(String text, int textSize, int textColor) ;
/**
* Set the title text.
* @param text
*/
public void setTitleText(String text) ;
/**
* Set the value of resource, size, and color of the Back button. Keep the default value if the value is null or 0.
* @param drawable
* @param size
* @param color
*/
public void setBackBtnInfo(Object drawable, int size, int color);
/**
* Set the resource of the Back button.
* @param drawable
*/
public void setBackBtnInfo(Object drawable);
/**
* Set the resource, size, and color of the left button. Keep the default value if the value is null or 0.
* @param drawable
* @param size
* @param color
* @param isText
*/
public void setLeftBtnInfo(Object drawable, int size, int color, boolean isText);
/**
* Set the resource of the left button.
* @param drawable
*/
public void setLeftButtonIcon(Drawable drawable);
public void setLeftButtonIcon(String unicode);
public void setLeftButtonText(String text);
/**
* Set the color and size of the left button.
* @param size
* @param color
* @param isText
*/
public void setLeftButtonFont(int size, int color, boolean isText);
/**
* Set the resource, size, and color of the right button. Keep the default value if the value is null or 0.
* @param drawable
* @param size
* @param color
* @param isText
*/
public void setRightBtnInfo(Object drawable, int size, int color, boolean isText) ;
/**
* Set the resource of the right button.
* @param drawable
*/
public void setRightButtonIcon(Drawable drawable);
public void setRightButtonIcon(String unicode);
public void setRightButtonText(String text);
/**
* Set the color and size of the right button.
* @param size
* @param color
* @param isText
*/
public void setRightButtonFont(int size, int color, boolean isText);
/**
* Make the progress bar start rotation.
*/
public void startProgressBar();
/**
* Make the progress bar stop and disappear.
*/
public void stopProgressBar() ;
/***
* Default processing of the gradual change during sliding. Use the default value for totalHeight.
* @param currentHeight The current height.
*/
public void handleScrollChange(int currentHeight);
/***
* Default processing of the gradual change during sliding.
*
* @param totalHeight The total height.
* @param currentHeight The current height.
*/
public void handleScrollChange(int totalHeight, int currentHeight) ;
/**
* Set the pattern color (white) with transparent background.
*/
public void setColorWhiteStyle();
/**
* Set the pattern color with white background.
*/
public void setColorOriginalStyle();
/**
* Make the Back button not displayed.
*/
public void setBackButtonGone();
/**
* Add a search box (input is not allowed) used only for visual adjustment.
* @param search
*/
public void setTitle2Search(String search);
/**
* Convert the search box into a title.
*/
public void setSearch2Title();
/**
* The font color in the search box is set to black and the background is set to white.
*/
public void setSearchColorOriginalStyle();
/**
* The font color in the search box is set to white and the background is set to transparent.
*/
public void setSearchColorTransStyle();
/**
* Add a red dot to the left icon.
* @param flagView
*/
public void attachFlagToLeftBtn(AUWidgetMsgFlag flagView);
/**
* Add a red dot to the right icon
* @param flagView
*/
public void attachFlagToRightBtn(AUWidgetMsgFlag flagView) ;
/**
* Add a red dot to the targetView.
* @param targetView
* @param flagView
*/
public void attachFlagView(AURelativeLayout container, View targetView, AUWidgetMsgFlag flagView;
Custom properties
Property | Description | Type |
---|---|---|
backgroundDrawable | The whole background of the title bar. | Reference |
backIconColor | The color of the back arrow. | Color, Reference |
titleText | Title text. | String, Reference |
titleTextSize | The font size of the title. | Dimension, Reference |
titleTextColor | The font color of the title. | Color, Reference |
leftIconResid | ID of the .png or .jpg image corresponding to the left icon. | Reference |
leftIconUnicode | The Unicode character of the left icon. | String, Reference |
leftIconColor | The color of the left icon. | Color, Reference |
leftIconSize | The size of the left icon. | Dimension, Reference |
leftText | Content of the left text. | String, Reference |
leftTextColor | The color of the left text. | Color, Reference |
leftTextSize | The size of the left text. | Dimension, Reference |
rightIconResid | ID of the .png or .jpg image corresponding to the right icon. | Reference |
rightIconUnicode | The Unicode character of the right icon. | String, Reference |
rightIconColor | The color of the right icon. | Color, Reference |
rightIconSize | The size of the right icon. | Dimension, Reference |
rightText | Content of the right text. | String, Reference |
rightTextColor | The color of the right text. | Color, Reference |
rightTextSize | The size of the right text. | Dimension, Reference |
Sample code
In the following sample code, the reference path of aui
is: xmlns:aui="http://schemas.android.com/apk/res/com.alipay.mobile.antui"
.
Basic usage
<com.alipay.mobile.antui.basic.AUTitleBar
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
aui:aui_titleText="Title"
aui:aui_titleTextSize="@dimen/AU_TEXTSIZE2"
aui:aui_titleTextColor="#f64219"
aui:leftIconUnicode="@string/iconfont_user_setting"
aui:rightText="Test2"/>

Transparent scrolling
<com.alipay.mobile.antui.basic.AUTitleBar
android:id="@+id/title_bar"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
aui:rightIconUnicode="@string/iconfont_user_setting"
aui:aui_titleText="Transparent Title Test" />
titleBar.handleScrollChange(testImg.getMeasuredHeight(), 0);
testScroll.setScrollViewListener(new AUScrollViewListener() {
@Override
public void onScrollChanged(ScrollView scrollView, int x, int y, int oldx, int oldy) {
titleBar.handleScrollChange(y);
}
});
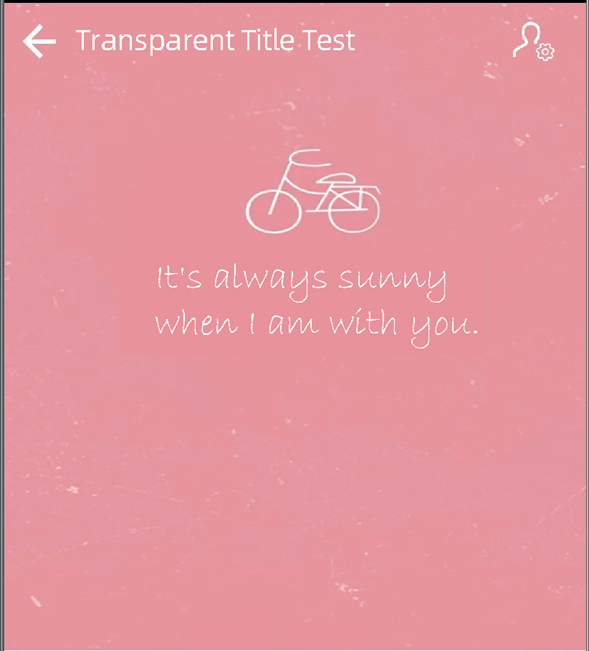
Title with a red dot
<com.alipay.mobile.antui.basic.AUTitleBar
android:id="@+id/progress_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
aui:aui_titleText="Refreshable Title"
aui:leftIconUnicode="@string/iconfont_scan"
aui:rightIconUnicode="@string/iconfont_more"/>
AUTitleBar processBar = (AUTitleBar) findViewById(R.id.progress_title);
processBar.startProgressBar();
WidgetMsgFlag j = new WidgetMsgFlag(this);
j.showMsgFlag();
processBar.attachFlagToLeftBtn(j);
WidgetMsgFlag i = new WidgetMsgFlag(this);
i.showMsgFlag(12);
processBar.attachFlagToRightBtn(i);
