This topic describes the AUInputBox, AUImageInputBox, and AUTextCodeInputBox input box components provided by mPaaS. The AUImageInputBox and AUTextCodeInputBox components inherit the AUInputBox component.
AUInputBox
The AUInputBox component contains the following:
An AUEditText text input box
A tag name displayed on the left of the input box
A Delete button that is displayed when an input box gets focus and the content is not empty
Sample image
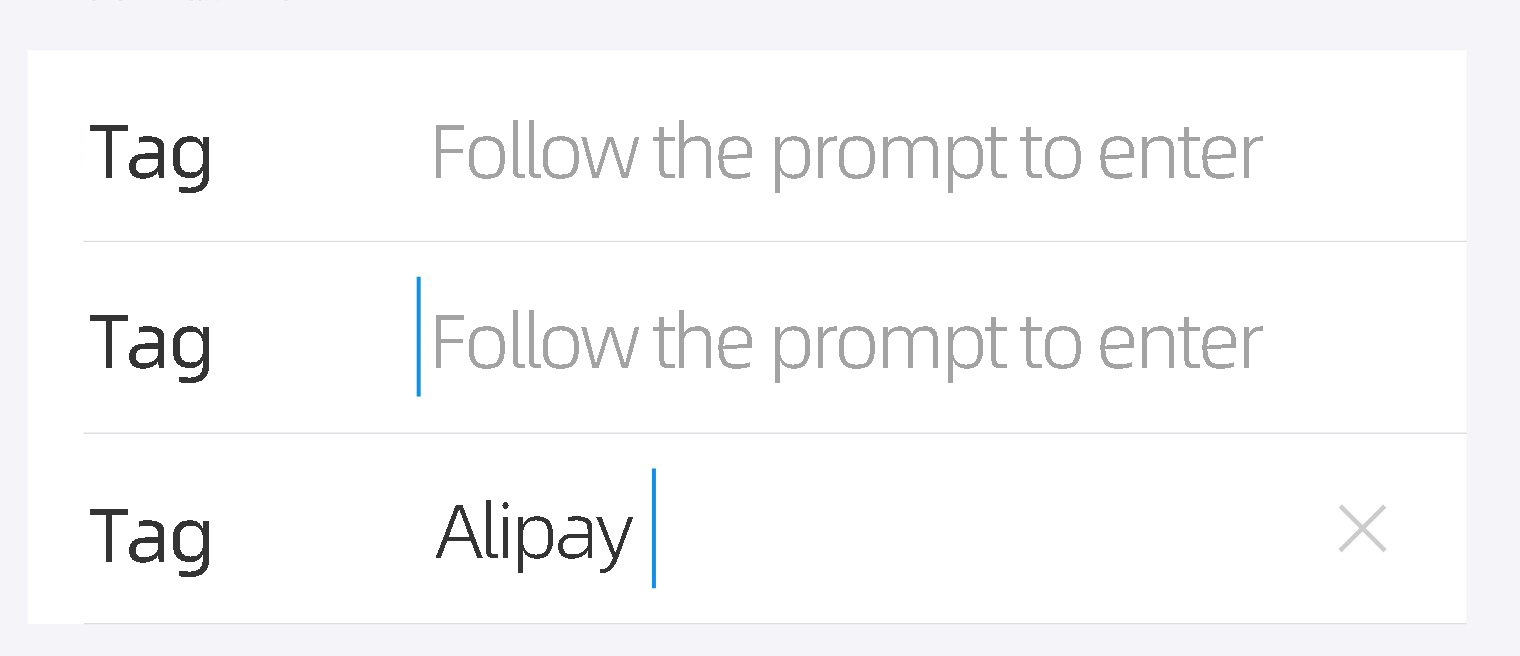
Dependency
See Quick start.
API description
/**
* Get the string after UBB encoding.
*/
public String getUbbStr()
/***
* Set the emoji font size, in pixels(px).
*/
public void setEmojiSize(int emojiSize)
/***
* Specify whether support to emoji.
*/
public void setSupportEmoji(boolean isSupport) {
this.supportEmoji = isSupport;
}
/**
* Set a Formatter to format the input.
* After the setting is completed, the text that has been entered does not take effect immediately. The effects will show upon subsequent text input.
*/
public void setTextFormatter(AUFormatter formatter)
/**
* Specify whether the input text is bold.
*
* @param isBold The value true indicates that the text is bold and the value false indicates that the text is normal.
*/
public void setApprerance(boolean isBold)
/**
* Set a special listener to be called when an action is performed on the
* text view. This will be called when the enter key is pressed, or when an
* action supplied to the IME is selected by the user. Setting this means
* that the normal hard key event will not insert a newline into the text
* view, even if it is multi-line; holding down the ALT modifier will,
* however, allow the user to insert a newline character.
*/
public void setOnEditorActionListener(OnEditorActionListener l)
/**
* Adds a TextWatcher to the list of those whose methods are called whenever
* this TextView's text changes.
*/
public void addTextChangedListener(TextWatcher watcher)
/**
* The Delete button will be displayed after entering text in the input box, and setting the object received by click event of the delete button here.
*/
public void setCleanButtonListener(View.OnClickListener listener)
/**
* Set the text content of the input box.
*/
public void setText(CharSequence inputContent)
/**
* Get the text content. If the text is formatted, the calling party needs to process the text to meet the required format.
*/
public String getInputedText()
/**
* The EditText control for getting the input box.
*/
public AUEditText getInputEdit()
/**
* Set the tag text.
* @param title The input tag text.
*/
public void setInputName(String title)
/**
* The control for getting the input content name (tag name).
*/
public AUTextView getInputName()
/**
* The font size of the input content name, in pixels(px).
*/
public void setInputNameTextSize(float textSize)
/**
* Set the font size of the input box, in pixels(px).
*/
public void setInputTextSize(float textSize)
/**
* The text color for the input content.
*/
public void setInputTextColor(int textColor)
/**
* Set the input content type.
*/
public void setInputType(int inputType)
/**
* Set prompt message.
*/
public void setHint(String hintString)
/**
* Set the left tag icon.
*/
public void setInputImage(Drawable drawable)
/**
* Get the left tag icon.
*/
public AUImageView getInputImage()
/**
* Set the color of the prompt message.
*/
public void setHintTextColor(int textColor)
/**
* Set the maximum input length of the input box.
*
* @param maxlength If the value is 0 or smaller, the length is not restricted.
*/
public void setMaxLength(int maxlength)
/**
* The control for getting the Clear button.
*/
public AUIconView getClearButton()
/**
* Get the setting of whether to show the Clear button.
*/
public boolean isNeedShowClearButton() {
return isNeedShowClearButton;
}
/**
* Specify whether to show the Clear button. If the parameter is set to false, the Clear button will not be showed in any case.
*/
public void setNeedShowClearButton(boolean isNeedShowClearButton)
/**
* Set the border style of the control, including upper, middle, lower, and independent.
* This method is in the AULineGroupItemInterface API.
* This method is automatically called when the control is used with LineGroupView.
*
* @param positionStyle Use the variables defined in AULineGroupItemInterface: TOP, CENTER, BOTTOM, NORMAL, LINE, and NONE.
*/
@Override
public void setItemPositionStyle(int positionStyle)
/**
* Get the input content type.
*/
public int getInputType()
Code sample
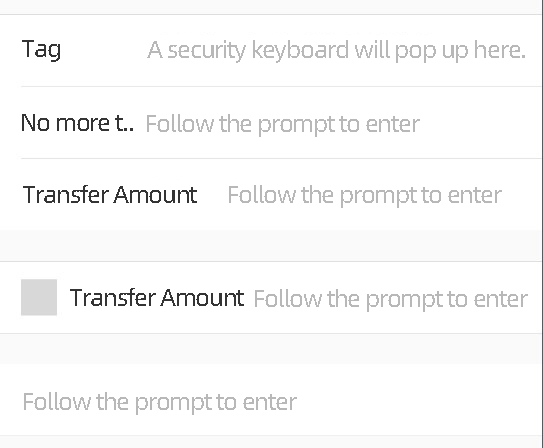
<com.alipay.mobile.antui.input.AUInputBox
android:id="@+id/safeInputBox"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:listItemType="top"
app:inputName="Tag 1"
app:inputType="textPassword"
app:inputHint="This input box pops up a security keyboard"/>
<com.alipay.mobile.antui.input.AUInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:listItemType="center"
app:inputName="Tag 2"
app:inputHint="Input as prompted"/>
<com.alipay.mobile.antui.input.AUInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:listItemType="bottom"
app:inputName="Transfer amount"
app:inputHint="Input as prompted"/>
<com.alipay.mobile.antui.input.AUInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
app:inputImage="@drawable/image"
app:inputName="Transfer amount"
app:inputHint="Input as prompted"/>
<com.alipay.mobile.antui.input.AUInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
app:inputHint="Input as prompted"/>
AUImageInputBox
AUImageInputBox inherits AUInputBox and contains the following:
A IconView on the right to show icons or Unicode
A TextView on the right
Sample image
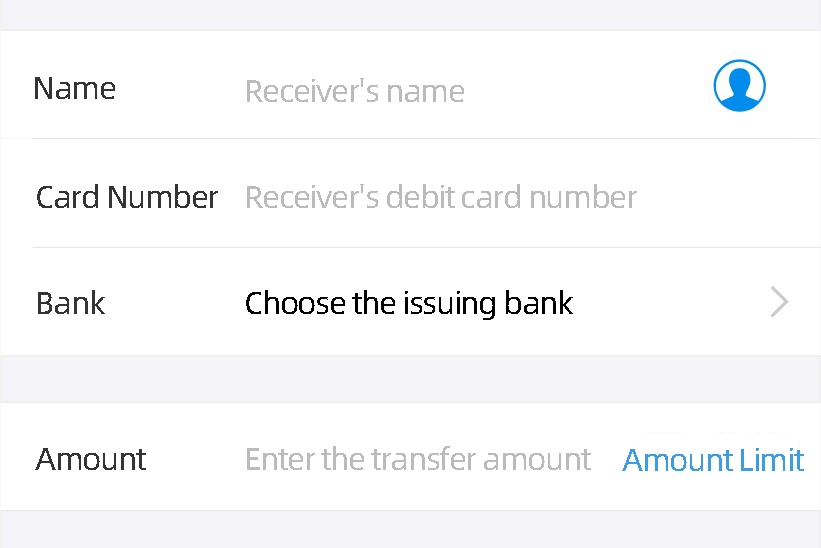
Dependency
See Quick start.
API description
/**
* Set the background of the rightmost functional button.
* If the background of the Set button is empty, the functional button will not be showed, which is consistent with the AUInputBox function.
*/
public void setLastImgDrawable(Drawable drawable)
/**
* Set the background of the rightmost functional button.
* @param unicode
*/
public void setLastImgUnicode(String unicode)
/**
* Specify whether the rightmost icon is visible.
* @param visible
*/
public void setLastImgBtnVisible(boolean visible)
/**
* Set listening on the rightmost functional button.
*/
public void setLastImgClickListener(View.OnClickListener l)
/**
* Set the rightmost text.
* @param lastText
*/
public void setLastTextView(String lastText)
/**
* Get the rightmost TextView.
*
* @return Get the rightmost TextView.
*/
public AUTextView getLastTextView()
/**
* Get the rightmost IconView.
* @return
*/
public AUIconView getLastImgBtn()
Code sample
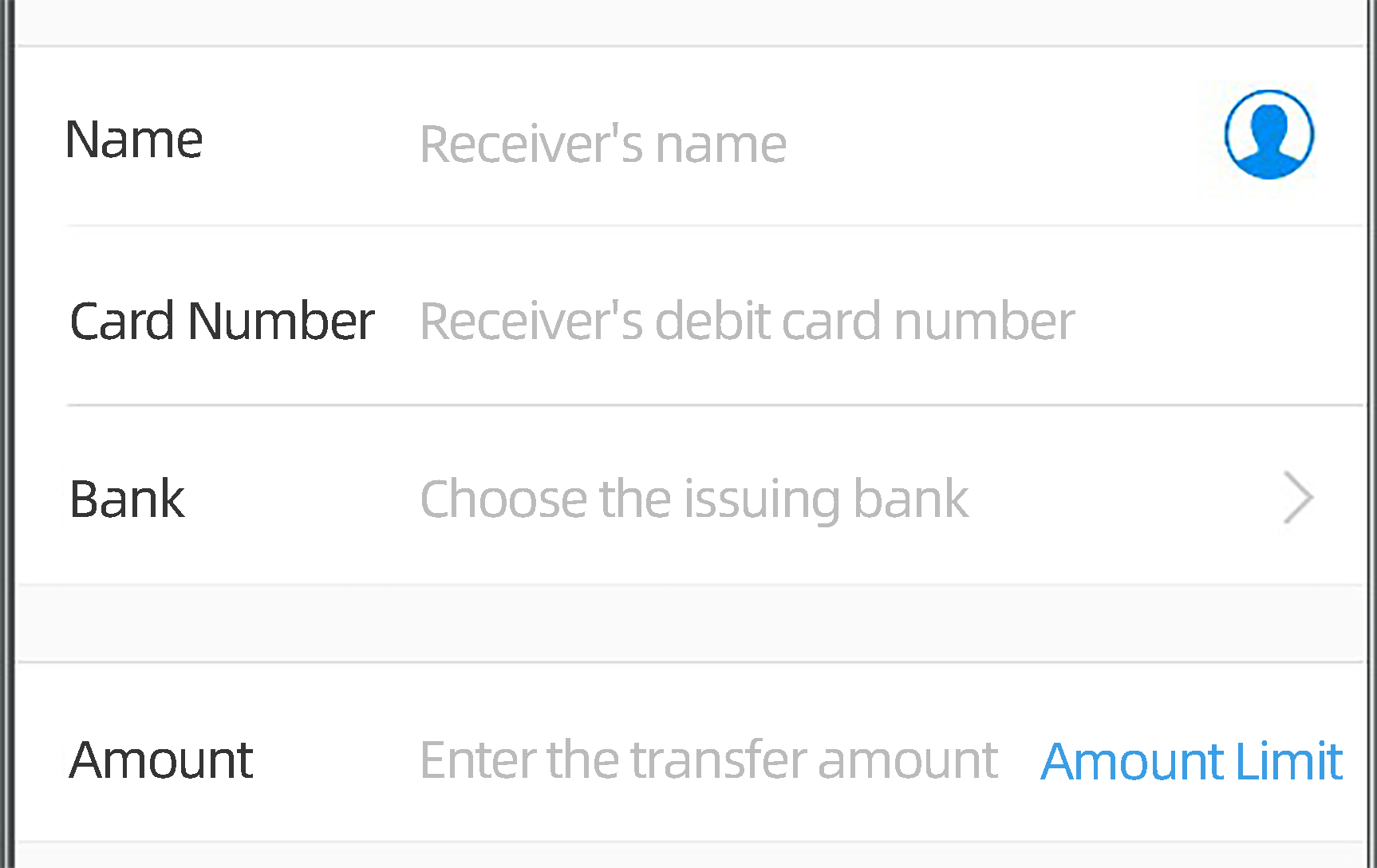
<com.alipay.mobile.antui.input.AUImageInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
app:listItemType="top"
app:inputName="Name"
app:inputHint="Payee name"
app:input_rightIconUnicode="@string/iconfont_phone_contact" />
<com.alipay.mobile.antui.input.AUImageInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:listItemType="center"
app:inputName="Card number"
app:inputHint="Savings card number of the payee"/>
<com.alipay.mobile.antui.input.AUImageInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:listItemType="bottom"
app:inputName="Bank"
app:inputHint="Select bank"
app:input_rightIconDrawable="@drawable/table_arrow" />
<com.alipay.mobile.antui.input.AUImageInputBox
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
app:inputName="Amount"
app:inputHint="Enter transfer amount"
app:input_rightText="Amount limit" />
AUTextCodeInputBox
AUTextCodeInputBox inherits AUInputBox and contains a text button used for sending the SMS verification code on the right.
Sample image

Dependency
See Quick start.
API description
/**
* Set the callback for the Send button clicking event.
* @param callback When a user clicks the Send button, the OnSendCallback.onSend() method will be called back.
*/
public void setOnSendCallback(OnSendCallback callback)
/**
* Reset the current time to zero.
*/
public void currentSecond2Zero()
/**
* Set the current time.
*/
public void setCurrentSecond(int current)
/**
* Get the current time.
*/
public int getCurrentSecond()
/**
* Get the Send button.
*/
public AUButton getSendCodeButton()
/**
* The release timer for business calling.
*/
public void releaseTimer()
/**
* Start countdown for the button.
*/
public void scheduleTimer()
public interface SendButtonEnableChecker {
public boolean checkIsEnabled();
}
/**
* This method sets the method used to detect whether SendButton is available.
* If this method detects that the button is unavailable, the button is dimmed when the updateSendButtonEnableStatus method is called.
* Otherwise, the button is available when the updateSendButtonEnableStatus method is called according to the countdown logic.
* The button is available only when all checks are available, or it will be dimmed.
*/
public void setSendButtonEnableChecker(SendButtonEnableChecker checker)
/**
* Update the SendButton availability state based on the internal state of SendButtonEnableChecker and CheckCodeSendBox.
* The button is available only when all checks are available, or it will be dimmed.
*/
public void updateSendButtonEnableStatus()
/**
* Get SendResultCallback.
*/
public SendResultCallback getSendResultCallback()
Code sample

XML
<com.alipay.mobile.antui.input.AUTextCodeInputBox
android:id="@+id/au_textcode_input"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"/>
Java
final AUTextCodeInputBox textCodeInputBox = (AUTextCodeInputBox) findViewById(R.id.au_textcode_input);
textCodeInputBox.setOnSendCallback(new OnSendCallback() {
@Override
public void onSend(final SendResultCallback callback) {
// The RPC request to the server for a verification code.
boolean resendSmsRpcSuccess = true;
if (resendSmsRpcSuccess) {
// Start countdown when the verification code is successfully sent.
callback.onSuccess();
// The verification code is received.
Toast.makeText(InputActivity.this, "Verification code received: 123456", Toast.LENGTH_SHORT)
.show();
textCodeInputBox.setText("123456");
Log.d(TAG, "Input verification code: " + textCodeInputBox.getInputedText());
} else {
// Failed to send the verification code. Enable the Send button again.
callback.onFail();
}
}
});
Custom properties
The following table describes custom attribute parameters of the three components.
Property | Description | Type |
---|---|---|
inputName | The input content name. | String, Reference |
inputHint | The prompt content of the input box. | String, Reference |
maxLength | The maximum length of the input content. | Integer |
inputType | The input content type. | Enum, including textNormal, textNumber, textDecimal, and textPassword |
inputImage | The image to the left of the input box. | Reference |
listItemType | The background type of an item. | Enum, including top, center, bottom, normal, line, and none |
input_rightIconUnicode | The right-side icon. | String, Reference |
input_rightIconDrawable | The right-side image. | Reference |
input_rightText | The right-side hyperlink text. | String, Reference |