Note: Since the JSON data transferred by JS cannot contain the data type, errors may occur due to the data type when the transferred data is converted into a dictionary at the Native layer. We recommend that precise numeric values be transferred as strings. For example, {"value":9.45}
will be converted into {"value":9.449999999999999}
at the Native layer and then uploaded to the server. Instead, using {"value":"9.45"}
can avoid such error.
RPC API usage instruction
AlipayJSBridge.call('rpc', {
operationType: 'alipay.client.xxxx',
requestData: [],
headers: {}
}, function(result) {
console.log(result);
});
Code sample
<h1>Click the button to initiate an RPC request.</h1>
<a href="javascript:void(0)" class="btn rpc">Initiate a request.</a><br/>
<a href="javascript:void(0)" class="btn rpcHeader">Initiate a request with a response header returned.</a>
<script>
function ready(callback) {
// Call JS Bridge if it has been injected.
if (window.AlipayJSBridge) {
callback && callback();
} else {
// Listen to the injection event if it has not been injected.
document.addEventListener('AlipayJSBridgeReady', callback, false);
}
}
ready(function() {
document.querySelector('.rpc').addEventListener('click', function() {
AlipayJSBridge.call('rpc', {
operationType: 'alipay.client.xxxx',
requestData: [],
headers: {}
}, function(result) {
alert(JSON.stringify(result));
});
});
document.querySelector('.rpcHeader').addEventListener('click', function() {
AlipayJSBridge.call('rpc', {
operationType: 'alipay.client.xxxx',
requestData: [],
headers: {},
getResponse: true
}, function(result) {
alert(JSON.stringify(result));
});
});
});
</script>
API
AlipayJSBridge.call('rpc', {
operationType:,
requestData:,
headers
}, fn);
Input parameters
Name | Type | Description | Required | Default value |
operationType | string | RPC service name. | Y | - |
requestData | array | Parameter of the RPC request. You need to build the parameter based on the specific RPC API. | N | - |
headers | object | Headers set for the RPC request. headers | N | {} |
gateway | string | Gateway address. | N | Alipay gateway |
compress | boolean | Whether request gzip compression is supported. | N | true |
disableLimitView | boolean | Whether to prevent the unified traffic-limiting window from popping up when the RPC gateway is subject to throttling. | N | false |
timeout | int | RPC timeout duration, in seconds. | N | Timeout durations are set by the framework in a unified manner and the policy is complex. Specifically, the timeout duration is set to 20s in a Wi-Fi environment for iOS and 30s in other environments. For Android, the timeout duration is set to a value between 12s and 42s in a Wi-Fi or 4G environment, and to a value between 32s and 60s in other environments. |
getResponse | boolean | Whether to obtain the RPC response header (note: if it is set to true, the response data is nested by one more layer and can be used to obtain the trace ID/entity ID during data backflow reporting). | N | false |
fn | function | Callback function. | N | - |
Output parameters
Parameter carried in the callback function: result: {error }
Name | Type | Description |
error | string | Error code |
Error code description
Error code | Description |
10 | Network error |
11 | Request timeout |
Others | Defined by the Mobile Gateway |
Native RPC error codes
Error code | Description |
1000 | Success |
0 | Unknown error |
1 | The client cannot find the communication object. |
2 | Network access is unavailable on the client (the error code is converted to 10 in JSAPI). |
3 | The client certificate is incorrect. |
4 | The network connection on the client times out. |
5 | The speed of the network connection on the client is too low. |
6 | The server does not give a response upon a request from the client. |
7 | A network IO error occurs on the client. |
8 | A network request scheduling error occurs on the client. |
9 | A processing error occurs on the client. |
10 | A data deserialization error occurs on the client, or the data format on the server is incorrect. |
11 | Logging in to the client failed. |
12 | The login account on the client is changed. |
13 | The request is interrupted. For example, the network request will be interrupted when the thread is interrupted. |
14 | A network cache error occurs on the client. |
15 | A network authorization error occurs on the client. |
16 | A DNS resolution error occurs. |
17 | operationType is not on the whitelist. |
1001 | The access is denied. |
1002 | The call times exceed the limit: The system is busy. Please try again later. |
2000 | Login has timed out. Please log in again. |
3000 | No operation type is present, or the operation type is not supported. |
3001 | The requested data is empty: The system is busy. Please try again later. |
3002 | The data format is incorrect. |
4001 | The service request has timed out. Please try again later. |
4002 | An exception occurs in remotely calling the service system: The network is busy. Please try again later. |
4003 | Creating a remote call agent failed: The network is busy. Please try again later. |
5000 | Unknown error: Sorry. Operations are not allowed for now. Please try again later. |
6000 | The RPC service is not found. |
6001 | the RPC target method is not found. |
6002 | The RPC parameter quantity is incorrect. |
6003 | The RPC target method is not accessible. |
6004 | An RPC JSON parsing exception occurs. |
6005 | RPC parameters are invalid when the target method is called. |
6666 | An exception occurs on the RPC service. |
7000 | No public key is set. |
7001 | The parameters for signature verification are insufficient. |
7002 | Signature verification failed. |
7003 | Signature verification timestamp check failed. |
7004 | The operationType parameter in the signature verification RPC API is empty. |
7005 | The productId parameter is empty. |
7006 | Signature verification API: The did parameter is empty. |
7007 | Signature verification API: The request sending time parameter t is empty. |
7008 | Signature verification API: The IMEI (client device identifier) parameter is empty. |
7009 | Signature verification API: The IMSI (client user identifier) parameter is empty. |
7010 | Signature verification API: The API version number is empty. |
7011 | Signature verification API: The user is not authorized. |
7012 | Signature verification API: The RPC API is not opened up. |
7013 | Signature verification API: The product ID is not registered, or no key is obtained. |
7014 | Signature verification API: The signature data is empty. |
7015 | Signature verification API: The subscription is invalid. |
7016 | Signature verification API: The transferred sid in the login request RPC API is empty. |
7017 | Signature verification API: The transferred sid in the login request RPC API is invalid. |
7018 | Signature verification API: The transferred token in the login request RPC API is invalid. |
7019 | Signature verification API: The alipayuserid obtained by the login request RPC API is empty. |
8001 | etag: The response data has no changes. |
RPC custom gateway
You can specify the gateway address to send request to in the RPC.
RPC throttling logic
Container version | disableLimitView | Action | Callback parameter |
<=9.9.5 | true | Silent | 1002 |
<=9.9.5 | false | Alert | 1002 |
>=9.9.6 | true | Silent | 1002 |
>=9.9.6 | false | Gateway handling | 100201 |
Action Type | Description |
Silent | None |
Alert | A unified throttling box is displayed, as shown in the following figure. |
Toast | A system toast is displayed. If the user exits the system, no toast is displayed. |
Gateway handling | The action is silent, alert, or toast, depending on the RPC configuration for the gateway. |
RPC throttling pop-up box
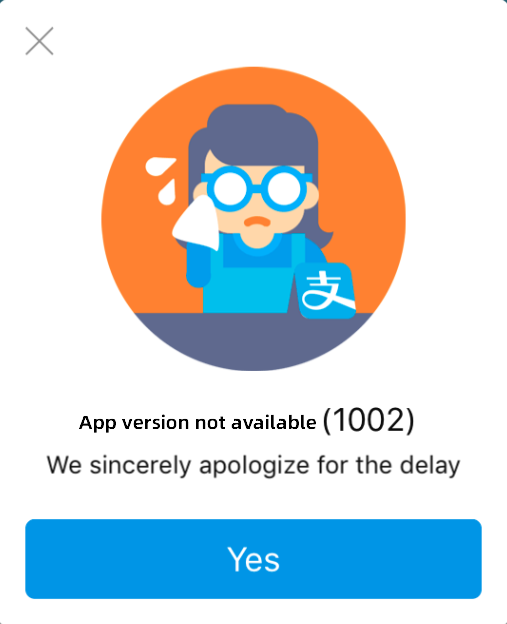
FAQ
Q: How to handle ESLint: 'AlipayJSBridge' is not defined
?
A: For questions not defined by AlipayJSBridge, try the following two solutions :
Solution 1:
window.AlipayJSBridge.call('rpc');
Solution 2:
const { AlipayJSBridge } = window; AlipayJSBridge.call('rpc');