This topic describes how to connect Java applications that use the Spring Boot framework to Identity as a Service (IDaaS).
For more information about how the connection works and the call process, see Connect self-developed applications to IDaaS for SSO.
IDaaS uses the OpenID Connect (OIDC) Authorization Code grant type to support connection to self-developed applications. This grant type is backward compatible with the OAuth 2.0 Authorization Code grant type, so you can use the OAuth toolkit spring-boot-starter-oauth2-client
to implement SSO.
This toolkit encapsulates all the code for authorization calls and id_token parsing in the OIDC Authorization Code flow.
1. Import the toolkit
The following example shows how to add the dependency spring-boot-starter-oauth2-client
to the pom.xml
file:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.1</version>
</parent>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-oauth2-client</artifactId>
</dependency>
</dependencies>
2. Configure IDaaS information
The following example shows how to configure the client_id
, client_secret
, and issuer
in the application.properties
file:
spring.security.oauth2.client.registration.aliyunidaas.client-id=app_***
spring.security.oauth2.client.registration.aliyunidaas.client-secret=CS***
spring.security.oauth2.client.provider.aliyunidaas.issuer-uri=<issuer>
The preceding information can be obtained after an application is created. For more information, see Connect self-developed applications to IDaaS for SSO.
3. Configure Spring Security to require OAuth authentication
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.anyRequest().authenticated()
.and()
.oauth2Login();
}
}
4. Obtain user information
The toolkit automatically completes all the authorization calls and id_token verification.
You can obtain the user information by injecting @AuthenticationPrincipal OAuth2User user
:
import org.springframework.security.core.annotation.AuthenticationPrincipal;
import org.springframework.security.oauth2.core.user.OAuth2User;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class SampleController {
@GetMapping("/user")
public OAuth2User user(@AuthenticationPrincipal OAuth2User user) {
return user;
}
}
You can output user information on the /user endpoint.
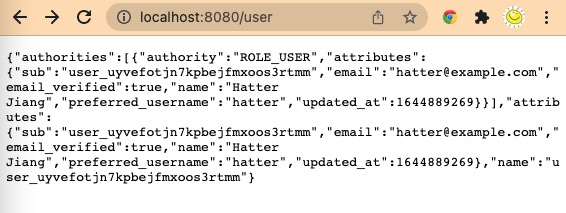
Use the user information to log the user on to complete the SSO process.
Deployment of an application behind a proxy
If you deploy an application behind a proxy, such as Alibaba Cloud Server Load Balancer (SLB) or NGINX, the following issues may occur during the test:
The user visits https://www.example.com.
The redirect_uri
generated when the user logs on to the application based on OIDC is changed to http://127.0.0.1:8080/oauth2/authorization/aliyunidaas. The logon request will fail.
To solve the issues, you must modify the logic of how Spring Boot generates a redirect_uri
by performing the following steps:
Add the following configuration item in Spring Boot:
server.forward-headers-strategy = NATIVE
If you use Alibaba Cloud SLB, you must select "Use the X-Forwarded-Proto header to retrieve the listener protocol" in the advanced configuration of SLB, as shown in the following figure.
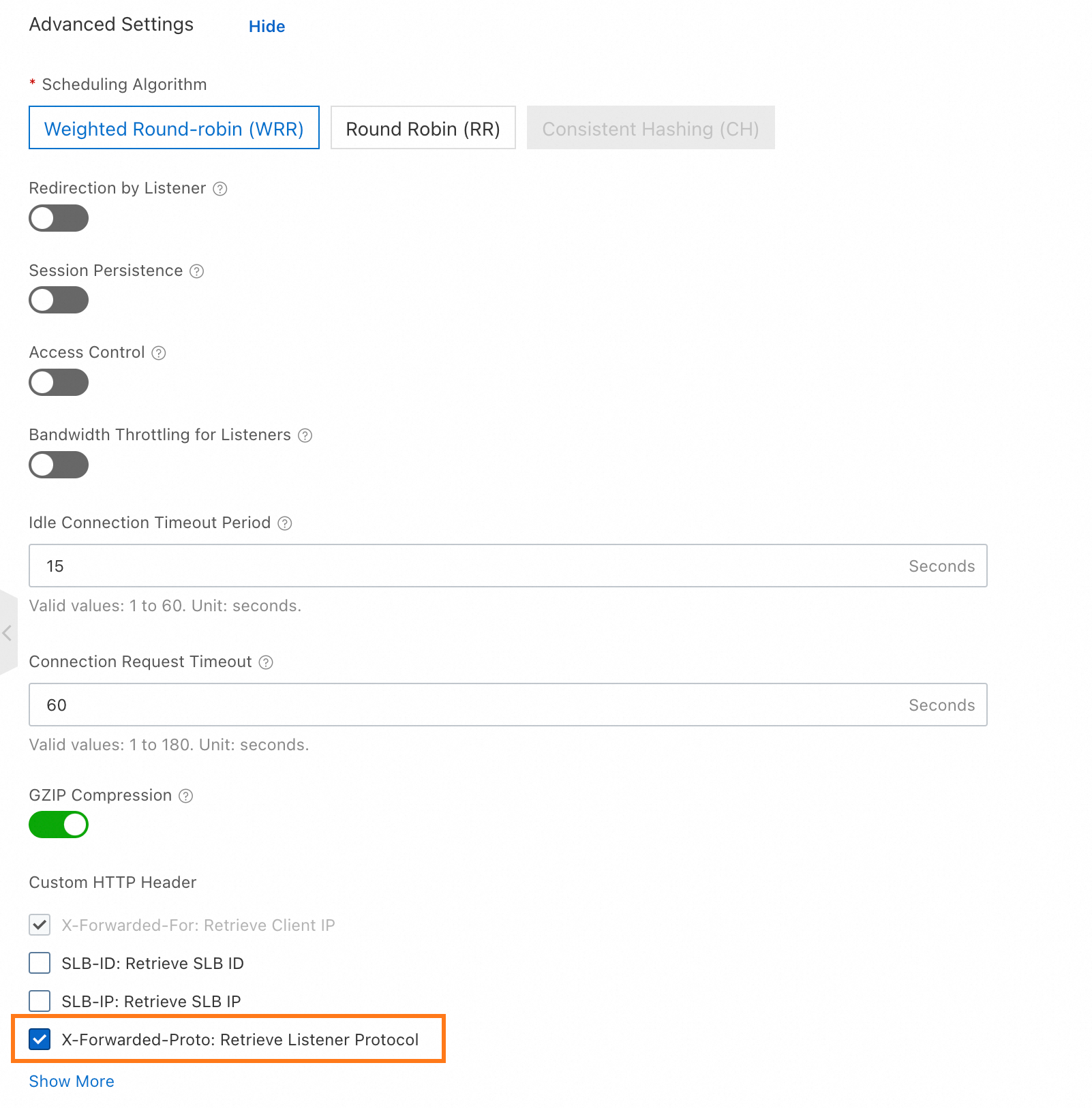
If you use an NGINX proxy between SLB and the application, you must configure the following parameters on the proxy:
proxy_set_header Host $host;
proxy_set_header X-Forwarded-Proto $proxy_x_forwarded_proto;
If you directly use an NGINX proxy to provide services over HTTPS, you must configure the following parameters:
proxy_set_header Host $host;
proxy_set_header X-Forwarded-Proto https;
If you use other proxy services or software, you need to check the configuration. Make sure that the correct HTTP headers Host
and X-forwarded-Proto
are specified in the request sent to Spring Boot.
References:
https://tools.ietf.org/html/rfc7239
https://docs.nginx.com/nginx/admin-guide/web-server/reverse-proxy/
https://docs.spring.io/spring-boot/docs/current/reference/html/howto.html#howto.webserver.use-behind-a-proxy-server