This topic provides a Hibernate connection example and tests several general features.
Configure dependencies
<dependency>
<groupId>com.oceanbase</groupId>
<artifactId>oceanbase-client</artifactId>
<version>2.4.0</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
<version>5.0.7.Final</version>
</dependency>
hibernate.cfg.xml
File content:
<?xml version='1.0' encoding='utf-8'?>
<!DOCTYPE hibernate-configuration PUBLIC
"-//Hibernate/Hibernate Configuration DTD 3.0//EN"
"http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd">
<hibernate-configuration>
<session-factory>
<!--OceanBase Database connection info-->
<property name="connection.driver_class">com.oceanbase.jdbc.Driver</property>
<property name="connection.url">jdbc:oceanbase://xxx.xxx.xxx.xxx:1521/</property>
<property name="connection.username">a****</property>
<property name="connection.password">******</property>
<!-- Optional configuration -->
<!-- Specifies whether to support dialects. -->
<!-- In Oracle mode, the following configuration is required. -->
<property name="dialect">org.hibernate.dialect.Oracle12cDialect</property>
<!-- Specifies whether to print SQL statements when create, read, update, and delete (CRUD) operations are performed. -->
<property name="show_sql">true</property>
<!--Automatic table creation (modification)-->
<!--<property name="hbm2ddl.auto">update</property>-->
<!--<property name="hbm2ddl.auto">create</property>-->
<!--Resource registration. Set the path of the entity class mapping file.)-->
<mapping resource="./UserModel.hbm.xml"/>
</session-factory>
</hibernate-configuration>
Test preparation
Entity class
public class User implements Serializable {
private Integer id;
private String username;
private Date birthday;
private String sex;
private String address;
//Comment out a field first to verify that the table is automatically modified according to the new properties of the entity class after the table is created based on the framework.
//private String tel;
//... The constructor and the get and set methods are omitted here.
}
Entity class mapping file in XML format
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE hibernate-mapping PUBLIC
'-//Hibernate/Hibernate Mapping DTD 3.0//EN'
'http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd'>
<hibernate-mapping>
<!-- The mapping between classes and tables is printed on the class element. -->
<!-- name: the full path class name. -->
<!-- table: the table name. -->
<class name="com.alibaba.ob.pojo.User" table="user">
<!-- The primary key mapping is printed on the id element. -->
<!-- name: the name of the attribute that serves as the primary key in the object. -->
<!-- column: the name of the primary key field in the table. -->
<!-- If the values of name and column are the same, the value of column can be ignored. -->
<id name="id" column="id">
<!--Set the class attribute of the generator element to "assigned". Manually create the generator element, and assign an ID for the class.-->
<generator class="identity" />
<!--**Pay attention to the strategy for generating the Hibernate primary key.**-->
</id>
<!-- The mapping between attributes and fields is printed on the property element. -->
<!-- name: the attribute name in the class. -->
<!-- column: the field name in the table. -->
<!-- If the values of name and column are the same, the value of column can be ignored. -->
<property name="username" />
<property name="birthday" />
<property name="sex" />
<property name="address"/>
<!--Same as the entity class-->
<!--<property name="tel"/>-->
</class>
</hibernate-mapping>
Sample code
Connection
@Test
public void testConnection(){
// Load the configuration information.
Configuration conf = new Configuration().configure();
// Create a SessionFactory object based on the configuration information.
SessionFactory sessionFactory = conf.buildSessionFactory();
// Open a session object related to the database.
Session session = sessionFactory.openSession();
System.out.println(session);
}
The connection is established.
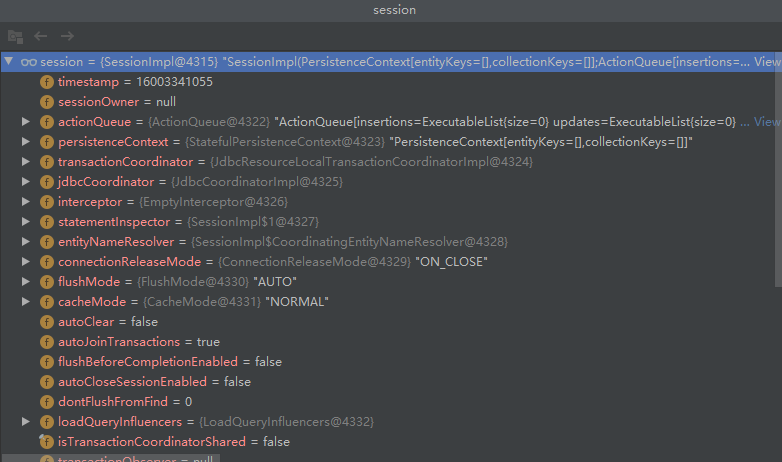
Automatic table creation
Open the row comment for the configuration file:

After you delete the user table in the database, perform the following test operations:
@Test
public void testCreateTableAndInsertData(){
// Create test objects.
User user = new User();
user.setUsername("hibernateTest");
user.setSex("Female");
user.setBirthday(new Date());
user.setAddress("Beijing");
//Start the transaction, where the statement data is obtained based on the session.
Transaction transaction = session.beginTransaction();
//Save data based on the session.
session.save(user);
//Commit the transaction.
transaction.commit();
// Close the session connection object after the operation is completed.
session.close();
}
View the executed SQL statements in the console and the statement execution results in OceanBase Database:

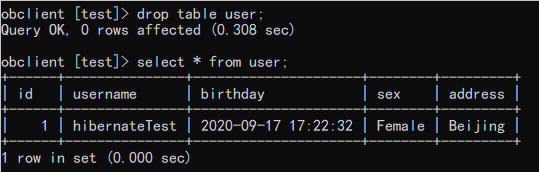
Test results show that OceanBase Database supports the Create Table feature of Hibernate.
Modify a table (add fields)
Modify the configuration file, as shown in the following example:
<!--Automatic table creation (modification)-->
<property name="hbm2ddl.auto">update</property>
<!--<property name="hbm2ddl.auto">create</property>-->
Open the comments on the tel attribute in the entity class and the XML file and execute the following method:
@Test
public void testAlterTable(){
// Create test objects.
User user = new User();
user.setUsername("hibernateTest");
user.setSex("Female");
user.setBirthday(new Date());
user.setAddress("Beijing");
user.setTel("12345678911");
//Start the transaction, where the statement data is obtained based on the session.
Transaction transaction = session.beginTransaction();
//Save data based on the session.
session.save(user);
//Commit the transaction.
transaction.commit();
// Close the session connection object after the operation is completed.
session.close();
}
The executed statements and statement execution results in the database are:

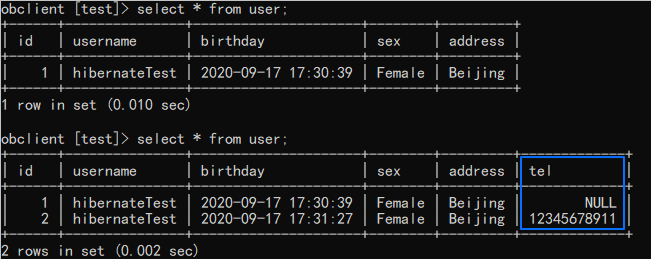
Based on the original table, the framework automatically adds fields to the table based on the entity class and the XML file.
Test results show that OceanBase Database supports the Alter Table feature of Hibernate.
Persist data
Test method:
@Test
public void testInsert() {
User u1 = new User("asd", new Date(), "Male", "Zhangzhou");
User u2 = new User("qwe", new Date(), "Female", "Hangzhou");
User u3 = new User("zxc", new Date(), "Male", "Shanghai");
User u4 = new User("xcv", new Date(), "Female", "Hangzhou");
User u5 = new User("sdf", new Date(), "Male", "Hangzhou");
User u6 = new User("wer", new Date(), "Female", "Hangzhou");
User u7 = new User("ert", new Date(), "Male", "Zhangzhou");
User u8 = new User("rty", new Date(), "Female", "Shanghai");
User u9 = new User("tyu", new Date(), "Male", "Hangzhou");
ArrayList<User> users = new ArrayList<>();
users.add(u1);
users.add(u2);
users.add(u3);
users.add(u4);
users.add(u5);
users.add(u6);
users.add(u7);
users.add(u8);
users.add(u9);
//Start the transaction, where the statement data is obtained based on the session.
Transaction transaction = session.beginTransaction();
//Save data based on the session.
for (User user : users) {
session.save(user);
}
//Commit the transaction.
transaction.commit();
// Close the session connection object after the operation is completed.
session.close();
}
Execution results:

Test results show that OceanBase Database supports the Insert feature and the primary key auto-increment feature of Hibernate.
Queries in HQL mode and SQL mode
Test method:
@Test
public void testQuery() {
//SQL mode
//SQLQuery query = sessionFactory.openSession().createSQLQuery("select * from user where sex = 'Male'").addEntity(User.class);
//HQL mode
//Query query = sessionFactory.openSession().createQuery("select User from User User where User.sex = 'Male'");
List<User> list = query.list();
list.forEach(System.out::println);
}
Execution results:
SQL mode:
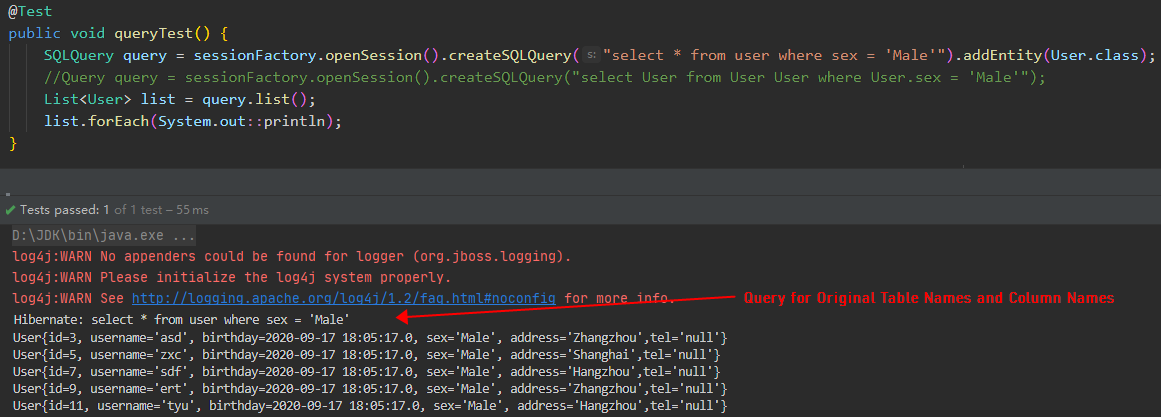
HQL mode:
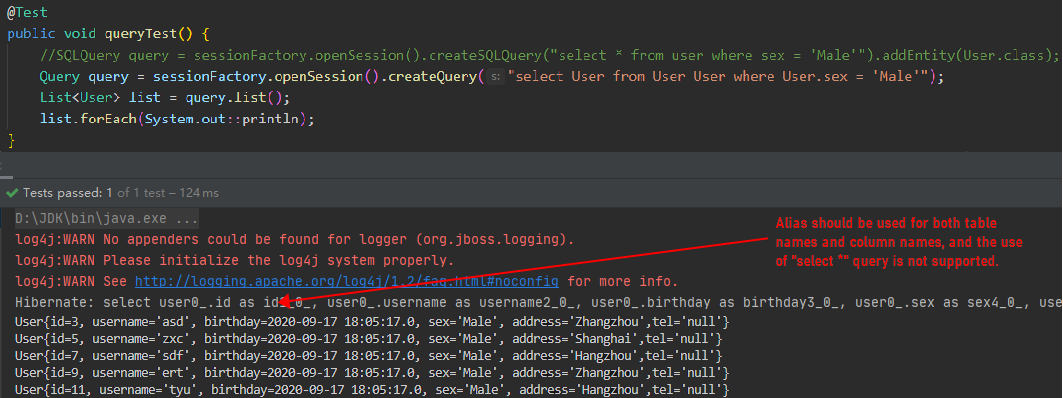
Test results show that OceanBase Database supports the Query feature of Hibernate.
Update
Test method:
@Test
public void testChange(){
User user = (User) session.createSQLQuery("select * from user where id = 1").addEntity(User.class).list().get(0);
System.out.println("Before modification: "+user);
user.setAddress("Hangzhou");
session.update(user);
user = (User) session.createSQLQuery("select * from user where id = 1").addEntity(User.class).list().get(0);
System.out.println("After modification: "+user);
}
Execution results:
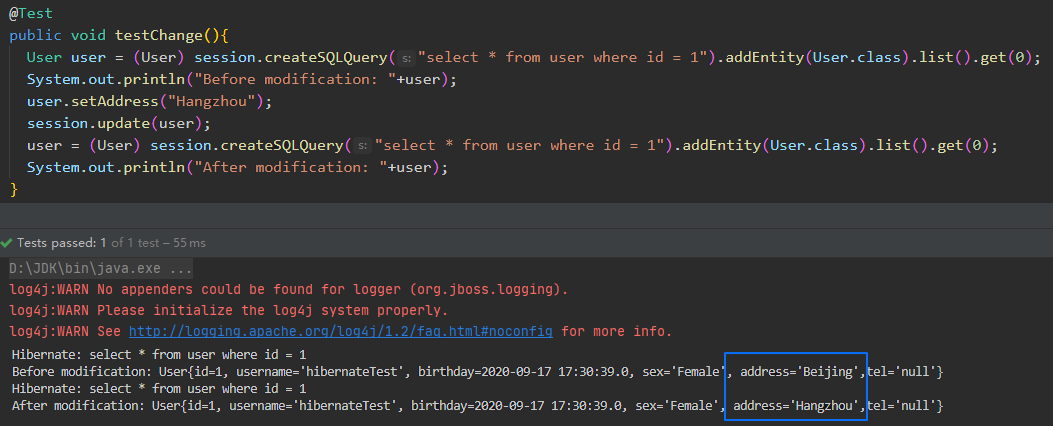
Test results show that OceanBase Database supports the Update feature of Hibernate.
Delete
Test method:
@Test
public void testDelete(){
Transaction transaction = session.beginTransaction();
List<User> list = session.createSQLQuery("select * from user").addEntity(User.class).list();
System.out.println(list);
int i = session.createSQLQuery("delete from user where id = " + list.get(0).getId()).executeUpdate();
list = session.createSQLQuery("select * from user").addEntity(User.class).list();
System.out.println(list);
//Commit the transaction.
transaction.commit();
// Close the session connection object after the operation is completed.
session.close();
}
Execution results:
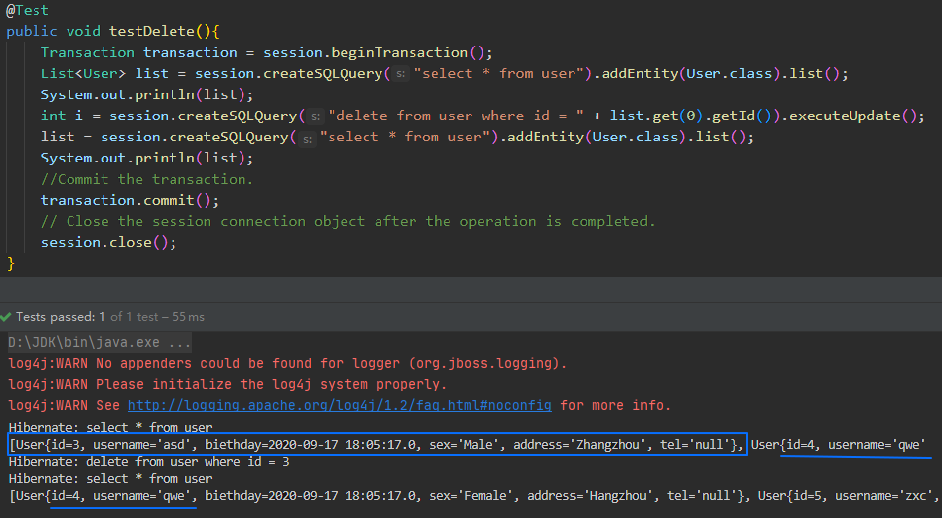
Test results show that OceanBase Database supports the Delete feature of Hibernate.