This topic describes the Flutter player with UI integration solution. By integrating AliPlayerWidget, you can quickly use the Flutter player.
Overview
AliPlayerWidget is a high-performance video playback widget designed for Flutter applications, built on the ApsaraVideo Player SDK flutter_aliplayer
. It supports video-on-demand (VOD), live streaming, list playback, and short drama scenarios. It also offers a rich set of features and highly flexible UI customization capabilities to meet the video playback needs of education, entertainment, E-commerce, and short drama applications.
Environment requirements
Category | Description |
Flutter version | No less than 2.10.0, recommended to use 3.22.2. |
JDK version | Recommended to use JDK 11. Note JDK 11 setup method: Preferences -> Build, Execution, Deployment -> Build Tools -> Gradle -> Gradle JDK -> select 11 (if 11 is not available, upgrade your Android Studio version) |
Android version | Supports Android 6.0 and above, Gradle version no less than 7.0. |
iOS version | Supports iOS 10.0 and above. |
Mobile phone processor | Architecture requirements: armeabi-v7a, arm64-v8a. |
Development tools | Recommended to use Android Studio or Visual Studio Code. |
Permission configuration
Network Permissions
License Configuration
Note
Note: If the License is not configured correctly, the player will not function properly and may throw a License authorization exception.
For more initialization configurations, refer to the aliplayer_widget_example
sample project.
Sample demo
To help developers quickly experience the features of AliPlayerWidget, we have built a demo package based on the aliplayer_widget_example
sample project. This demo package can be directly installed on the device and run without additional development environment configuration.
Acquisition method
Use your mobile phone to scan the following QR code to quickly download and install the demo package:
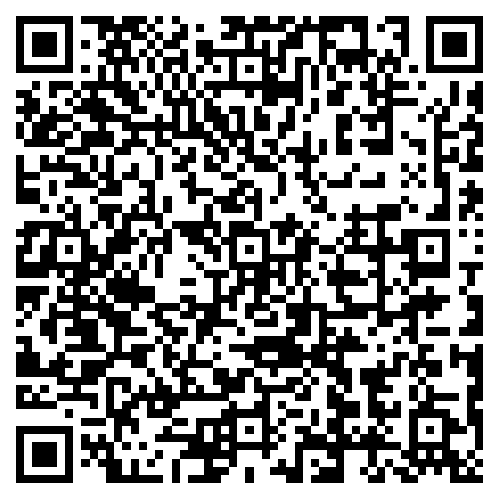
Note
Note: The QR code link points to the latest version of the demo package. Please ensure that your device has enabled the permission to install third-party applications.
SDK integration and download
aliplayer_widget is open-source and provides multiple download methods. If you need to customize the component, you can use the source code dependency method to directly obtain and modify the source code. The complete source code can be obtained in the following ways:
Download description | Download address |
Download description | Download address |
Pub source | Note aliplayer_widget recommends developers to integrate through package management tools |
|
GitHub | GitHub address |
Alibaba Cloud address | aliplayer_widget |
The download package includes:
Core Components: Complete implementation of AliPlayerWidget
and AliPlayerWidgetController
.
Sample Project: aliplayer_widget_example
provides code examples for VOD, live streaming, and list playback scenarios to help developers quickly integrate and use.
Documentation and Comments: The source code contains detailed comments and development guides for custom development and customization.
Integration method
Manually Add Dependencies
Add the following dependencies to your pubspec.yaml
file:
dependencies:
aliplayer_widget: ^x.y.z
Note
Note: x.y.z
represents the version number of aliplayer_widget
. You can check the latest stable version number on the Pub.dev official page and replace it with the actual value (for example, ^7.0.0
).
Use Command Line Tools
If you prefer to use the command line, you can directly run the following command to add dependencies:
flutter pub add aliplayer_widget
This command will automatically update your pubspec.yaml
file.
After adding dependencies, run the following command in the terminal to install the dependencies:
Through the above steps, AliPlayerWidget
has been successfully integrated into your project, and you can start using it!
Getting Started
Implement video playback
The following is a complete example showing how to embed a video player in a page. You can implement the video playback function with just a few lines of code.
Expand to View Sample Code
import 'package:flutter/material.dart';
import 'package:aliplayer_widget/aliplayer_widget_lib.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: VideoPlayerPage(),
);
}
}
class VideoPlayerPage extends StatefulWidget {
@override
_VideoPlayerPageState createState() => _VideoPlayerPageState();
}
class _VideoPlayerPageState extends State<VideoPlayerPage> {
late AliPlayerWidgetController _controller;
@override
void initState() {
super.initState();
_controller = AliPlayerWidgetController(context);
final data = AliPlayerWidgetData(
videoUrl: "https://example.com/video.mp4",
coverUrl: "https://example.com/cover.jpg",
videoTitle: "Sample Video",
);
_controller.configure(data);
}
@override
void dispose() {
_controller.destroy();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: AliPlayerWidget(
_controller,
),
),
);
}
}
Step Analysis
Initialize AliPlayerWidgetController
Create the _controller
instance in the initState
method.
Invoke configure
interface
Use AliPlayerWidgetData
to configure video URL, cover image, title, and other information.
Invoke _controller.configure(data)
to pass the data to the player widget.
Release Resources
In the dispose
method, invoke _controller.destroy()
to avoid memory leaks.
More Scenario Support
The above example demonstrates the basic usage of the VOD scenario. For more complex scenarios such as live streaming and list playback, refer to the aliplayer_widget_example
sample project, which contains detailed code and usage instructions.
Sample project
Project description
aliplayer_widget_example
is a sample project based on the aliplayer_widget
Flutter library, designed to help developers quickly get started and deeply understand how to integrate and use AliPlayerWidget
in actual projects.
Through this sample project, you can learn the following:
How to embed the video player widget.
How to configure playback functions for different scenarios (such as VOD, live streaming, and list playback).
How to use custom options to achieve a personalized user experience.
Compile and run
Run from the command line
Run from IDE
Clone the Repository
First, clone the aliplayer_widget_example
repository locally:
cd aliplayer_widget_example
Install Dependencies
Run the following command to install the dependencies required by the project:
Compile the Project
Compile the Android project
Compile the iOS project
If you need to compile the Android project, follow these steps:
Ensure that Android SDK and Gradle are installed.
Run the following command to generate the APK file:
After compilation, the APK file will be located at build/app/outputs/flutter-apk/app-release.apk
.
If you need to compile the iOS project, follow these steps:
Ensure that Xcode and CocoaPods are installed.
Initialize CocoaPods dependencies:
cd ios && pod install && cd ..
Run the following command to generate the iOS application package (IPA file):
After compilation, the IPA file will be located at build/ios/ipa/Runner.ipa
.
Run the Sample
Run the Android sample
flutter run lib/main.dart
Run the iOS sample
cd ios && pod install && cd ..//Run pod install before running
flutter run lib/main.dart
Use Android Studio
Open the project:
Launch Android Studio. Select Open an Existing Project
, then navigate to the cloned aliplayer_widget_example
directory and open it.
Install dependencies:
In Android Studio, click the pubspec.yaml
file, then click the Pub Get
button in the upper right corner to install dependencies.
Configure the device:
Ensure that an Android physical device is connected.
Run the application:
Click the green run button (Run
) in the toolbar, select the target device, and start the application.
Use VS Code
Open the project:
Launch VS Code. Select File -> Open Folder
, then navigate to the cloned aliplayer_widget_example
directory and open it.
Install dependencies:
Open the terminal (Ctrl + ~
), run the following command to install dependencies:
Configure the device:
Ensure that an Android or iOS physical device is connected. Use the device selector in VS Code (bottom left corner) to select the target device.
Run the application:
Press F5
or click the Run and Debug
icon in the left activity bar, select the Flutter
configuration, and start the debug session.
Use Xcode (iOS only)
Open the project:
Navigate to the ios
directory, double-click the Runner.xcworkspace
file to open the project in Xcode.
Install CocoaPods dependencies:
If CocoaPods dependencies are not yet installed, run the following command in the terminal:
cd ios && pod install && cd ..
Configure signing:
In Xcode, select the Runner
project, go to the Signing & Capabilities
tab, and configure a valid developer account and signing certificate.
Run the application:
Click the run button (▶️
) in the Xcode toolbar, select the target device, and start the application.
Sample description
The aliplayer_widget_example
project covers multiple typical scenarios, demonstrating the core features of AliPlayerWidget
and its practical applications in different scenarios. Whether it's a basic VOD playback page, a complex live streaming playback page, or a short video list playback page, developers can quickly master the integration and usage methods through this sample project and customize development according to their needs.
VOD playback page (LongVideoPage)
Live streaming playback page (LivePage)
Feature Description
This page demonstrates how to use AliPlayerWidget
to implement basic VOD video playback functionality.
Running Effect
After the page loads, the specified VOD video is automatically played, and the cover image and title are displayed. Users can pause, fast forward, and adjust the volume through the control bar. The example is as follows:
@override
void initState() {
super.initState();
_controller = AliPlayerWidgetController(context);
final data = AliPlayerWidgetData(
videoUrl: "https://example.com/sample-video.mp4",
coverUrl: "https://example.com/sample-cover.jpg",
videoTitle: "Sample Video Title",
);
_controller.configure(data);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: AliPlayerWidget(_controller),
);
}
Feature Description
This page demonstrates how to configure AliPlayerWidget
to support real-time live streaming media playback.
Running Effect
After the page loads, the live stream is played in real-time, supporting low-latency playback. Users can view messages in the chat window and send their comments. The example is as follows:
@override
void initState() {
super.initState();
_controller = AliPlayerWidgetController(context);
final data = AliPlayerWidgetData(
videoUrl: "https://example.com/live-stream.m3u8",
sceneType: SceneType.live,
);
_controller.configure(data);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: [
Expanded(child: AliPlayerWidget(_controller)),
_buildChatArea(),
_buildMessageInput(),
],
),
);
}
For the usage of short video list operations, see Integrate Micro-Short Drama Solution - Flutter.
Core components
AliPlayerWidget
is the core player component used to embed in Flutter applications and play videos.
Constructor
AliPlayerWidget(
AliPlayerWidgetController controller, {
Key? key,
List<Widget> overlays = const [],
});
Name | Description |
controller | Player controller used to manage playback logic. |
overlays | Optional list of overlay widgets for adding custom UI on top of the player component. |
Example is as follows
Expand to View Sample Code
AliPlayerWidget(
_controller,
overlays: [
Positioned(
right: 16,
bottom: 80,
child: Column(
children: [
IconButton(icon: Icon(Icons.favorite), onPressed: () {}),
IconButton(icon: Icon(Icons.share), onPressed: () {}),
],
),
),
],
);
AliPlayerWidgetController
is the core controller of the player component, used to manage the initialization, playback, and destruction logic of the player component.
Method Description
Name | Description |
configure(AliPlayerWidgetData data) | Configure the data source. |
play() | Start video playback. |
pause() | Pause playback. |
seek(Duration position) | Jump to the specified playback position. |
setUrl(String url) | Set the video playback URL. |
destroy() | Destroy the player instance and release resources. |
Example is as follows
final controller = AliPlayerWidgetController(context);
AliPlayerWidgetData data = AliPlayerWidgetData(
videoUrl: "https://example.com/video.mp4",
);
controller.configure(data);
controller.destroy();
AliPlayerWidgetData
is the data model required by the player component, including video URL, cover image, title, and other information.
Attribute Description
Name | Description |
videoUrl | Video playback URL (required). |
coverUrl | Cover image URL (optional). |
videoTitle | Video title (optional). |
thumbnailUrl | Thumbnail URL (optional). |
sceneType | Playback scenario type, default is VOD (SceneType.vod ). |
Example is as follows
AliPlayerWidgetData(
videoUrl: "https://example.com/video.mp4",
coverUrl: "https://example.com/cover.jpg",
videoTitle: "Sample Video",
sceneType: SceneType.vod,
);
Custom features
Overlay components
Through the overlays
parameter, you can easily overlay custom UI components on the player, such as adding like, comment, and share buttons.
AliPlayerWidget(
_controller,
overlays: [
Positioned(
right: 16,
bottom: 80,
child: Column(
children: [
IconButton(icon: Icon(Icons.favorite), onPressed: () {}),
IconButton(icon: Icon(Icons.comment), onPressed: () {}),
IconButton(icon: Icon(Icons.share), onPressed: () {}),
],
),
),
],
);
Common interfaces
AliPlayerWidget
provides a series of external interfaces to allow developers to directly control the player's behavior. These interfaces are exposed through AliPlayerWidgetController
and support playback control, status query, data update, and other functions. The following are some common external interfaces and their usage scenarios:
Configuration and Initialization
Name | Function description |
configure | Configure the player data source and initialize the player. |
prepare | Prepare the player (manually invoke the preparation process). |
Playback Control
Name | Function description |
play | Start video playback. |
pause | Pause playback. |
stop | Stop playback and reset the player. |
seek | Jump to the specified playback position. |
togglePlayState | Toggle playback state (play/pause). |
replay | Replay the video (usually used to restart after playback is complete). |
Playback Attribute Settings
Name | Function description |
setSpeed | Set playback speed. |
setVolume | Set the volume. |
setVolumeWithDelta | Adjust the volume based on the increment. |
setBrightness | Set screen brightness. |
setBrightnessWithDelta | Adjust screen brightness based on the increment. |
setLoop | Set whether to loop playback. |
setMute | Set whether to mute. |
setMirrorMode | Set mirror mode (such as horizontal mirror, vertical mirror, etc.). |
setRotateMode | Set the rotation angle (such as 90°, 180°, etc.). |
setScaleMode | Set the rendering fill mode (such as stretch, crop, etc.). |
selectTrack | Switch playback resolution. |
Other Settings
Name | Function description |
requestThumbnailBitmap | Request a thumbnail at a specified time point. |
clearCaches | Clear player caches (including video cache and image cache). |
getWidgetVersion | Obtain the version number of the current Flutter widget. |
Event notification
AliPlayerWidgetController
provides a series of ValueNotifier
to notify real-time changes in the player's status and user operations. The following are some commonly used notifiers
and their purposes:
Common notifier overview
Playback Status Management
Name | Function description |
playStateNotifier | Used to monitor changes in playback status (such as play, pause, stop, etc.). |
playErrorNotifier | Monitor errors that occur during playback, providing error codes and error descriptions. |
Playback Progress Management
Name | Function description |
currentPositionNotifier | Update the current playback progress in real-time (unit: milliseconds). |
bufferedPositionNotifier | Track the buffering progress of the video to help users understand the range of cached content. |
totalDurationNotifier | Provide the total duration of the video to facilitate calculating the playback progress percentage or displaying the total duration. |
Volume and Brightness Control
Notifier | Function description |
Notifier | Function description |
volumeNotifier | Monitor volume changes and support dynamic volume adjustment (range is usually 0.0 to 1.0). |
brightnessNotifier | Monitor screen brightness changes, allowing users to adjust brightness according to ambient light (range is usually 0.0 to 1.0). |
Resolution and Thumbnail
Notifier | Function description |
Notifier | Function description |
currentTrackInfoNotifier | Track changes in current video resolution information (such as standard definition, high definition, ultra-high definition, etc.) and provide details of the switched resolution. |
thumbnailNotifier | Monitor the loading status of thumbnails to ensure that the corresponding thumbnail preview can be displayed in real-time when dragging the progress bar. |
Usage method
By using ValueListenableBuilder
to monitor changes in notifier
, you can update the UI or execute logic in real-time. The example is as follows:
ValueListenableBuilder<Map<int?, String?>?>(
valueListenable: _controller.playErrorNotifier,
builder: (context, error, _) {
if (error != null) {
final errorCode = error.keys.firstOrNull;
final errorMsg = error.values.firstOrNull;
return Text("Playback error: [$errorCode] $errorMsg");
}
return SizedBox.shrink();
},
);
Features
Multi-scenario adaptation
Supports VOD, live streaming, list playback, and full-screen playback scenarios.
Rich feature set
Basic Features: Provides core features such as playback control, settings panel, cover image display, and resolution switching to meet regular video playback needs.
Gesture Control: Supports gesture adjustment of brightness, volume, and playback progress, providing intuitive and convenient operations to enhance user experience.
Flexible UI customization
Overlay Support: Supports custom overlay components with strong extensibility, allowing developers to implement complex features such as ads and live comments.
Modular Design: Built-in reusable UI components such as top bar, bottom bar, and settings panel, making it easy for developers to customize as needed.
Full-Screen Adaptation: Automatically adapts to horizontal and vertical screen switching to ensure the best display effect on different devices.
Event and status management
Real-Time Status Monitoring: Provides real-time data updates such as playback status, video size, and buffering progress to facilitate dynamic interaction by developers.
Event Callback Mechanism: Provides comprehensive player event monitoring, including playback start, pause, and completion status management, making it easy for developers to handle various playback scenarios.
Log and Debug Support: Built-in detailed logging system to facilitate troubleshooting and performance optimization.
Core advantages
Through simple API calls, developers can quickly integrate high-quality video playback features, significantly reducing development costs. Whether it's basic playback control or complex interactive scenarios such as gesture adjustment and overlay stacking, AliPlayerWidget can easily handle it, helping developers create a smooth and efficient user experience.
Easy to use
Complex video playback features can be implemented through simple API calls and quickly integrated into Flutter projects.
Flexible and extensible
Modular design supports various customization options, making it easy for developers to customize according to their needs.
Automatically adapts to different screen sizes and resolutions to ensure a consistent user experience.
High performance and stability
Based on the ApsaraVideo Player SDK, it provides a low-latency and high-stability video playback experience.
Optimized architecture design ensures efficient operation and smooth user experience through lightweight components, asynchronous loading, and event-driven models.
Cross-platform support
Fully utilizes Flutter's cross-platform features, supporting both Android and iOS platforms, enabling dual-end operation with a single development.
Precautions
Resource Release
When the page is destroyed, be sure to call the AliPlayerWidgetController.destroy()
method to release player resources.
Network Permissions
Ensure that the application has configured the necessary network permissions to load online videos.
Thumbnail Support
If you need to use the thumbnail feature, make sure to provide a valid thumbnail URL.
Debugging and Optimization
It is recommended to enable the logging feature during development to facilitate troubleshooting. Also, pay attention to optimizing the performance of overlay components to avoid affecting playback smoothness.
FAQ
For more common questions and troubleshooting suggestions about using the ApsaraVideo Player SDK, see Player FAQ.