Key Management Service (KMS) allows you to create keys and use the keys to encrypt and decrypt data in an efficient manner. This helps ensure the security of your data. This topic describes how to create and use a key.
Overview
KMS provides software-protected keys, hardware-protected keys, and default keys to meet your business, security, and compliance requirements. For more information, see Overview of key management and Key types and specifications.
KMS provides default keys free of charge. You can use default keys to perform server-side encryption in Alibaba Cloud services such as Elastic Compute Service (ECS). Default keys support only symmetric decryption. Default keys do not support client-side data encryption.
Software-protected keys support server-side encryption in Alibaba Cloud services, can be used to build application-layer cryptographic solutions, and provide API operations for client-side data encryption and decryption, and signing and verification. Software-protected keys support symmetric keys, asymmetric keys, and key rotation to reduce the risk of key leaks.
Hardware-protected keys support server-side encryption in Alibaba Cloud services, can be used to build application-layer cryptographic solutions, and provide API operations for client-side data encryption and decryption, and signing and verification. Hardware-protected keys support symmetric keys and asymmetric keys and provide more key specifications than software-protected keys. You can store hardware-protected keys in hardware security modules (HSMs) to meet the cryptographic requirements and comply with Federal Information Processing Standards (FIPS). Hardware-protected keys do not support key rotation.
Server-side encryption in Alibaba Cloud services
Keys can be used for server-side encryption in Alibaba Cloud services such as ECS to solve the security risk of plaintext data transmission on the server side. Key types that support this feature are software-protected keys, hardware-protected keys, and default keys.
Example
In this example, the default key of the customer master key (CMK) type is integrated into ECS. For more information about how to integrate Alibaba Cloud services with KMS, see Integration with KMS and Alibaba Cloud services that can be integrated with KMS.
Step 1: Enable the default key of the CMK type
Log on to the KMS console. In the top navigation bar, select a region. In the left-side navigation pane, choose .
On the Keys page, click Default Key. Find the default key of the CMK type that you want to enable and click Enable in the Actions column. Set the key name to alias/main.
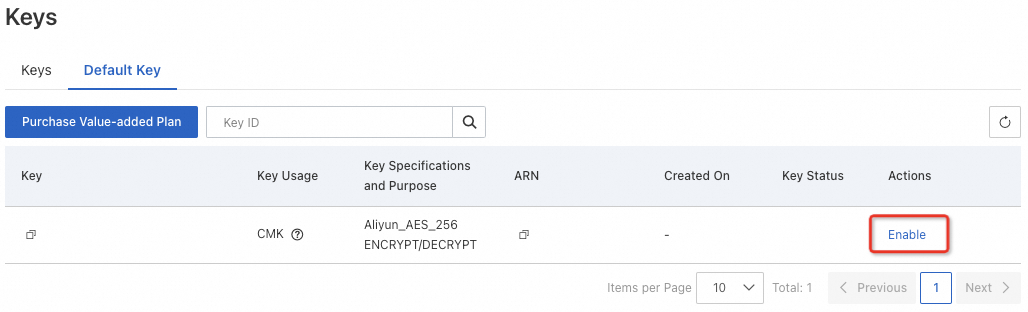
Note You can use the default key of the CMK type only for server-side encryption in Alibaba Cloud services, but not for client-side data encryption. If you want to implement client-side data encryption, create an instance and then create a software-protected key or a hardware-protected key.
Step 2: Integrate the default key of KMS into ECS
When you purchase an ECS instance on the buy page, select the default key named alias/main to enable server-side encryption.
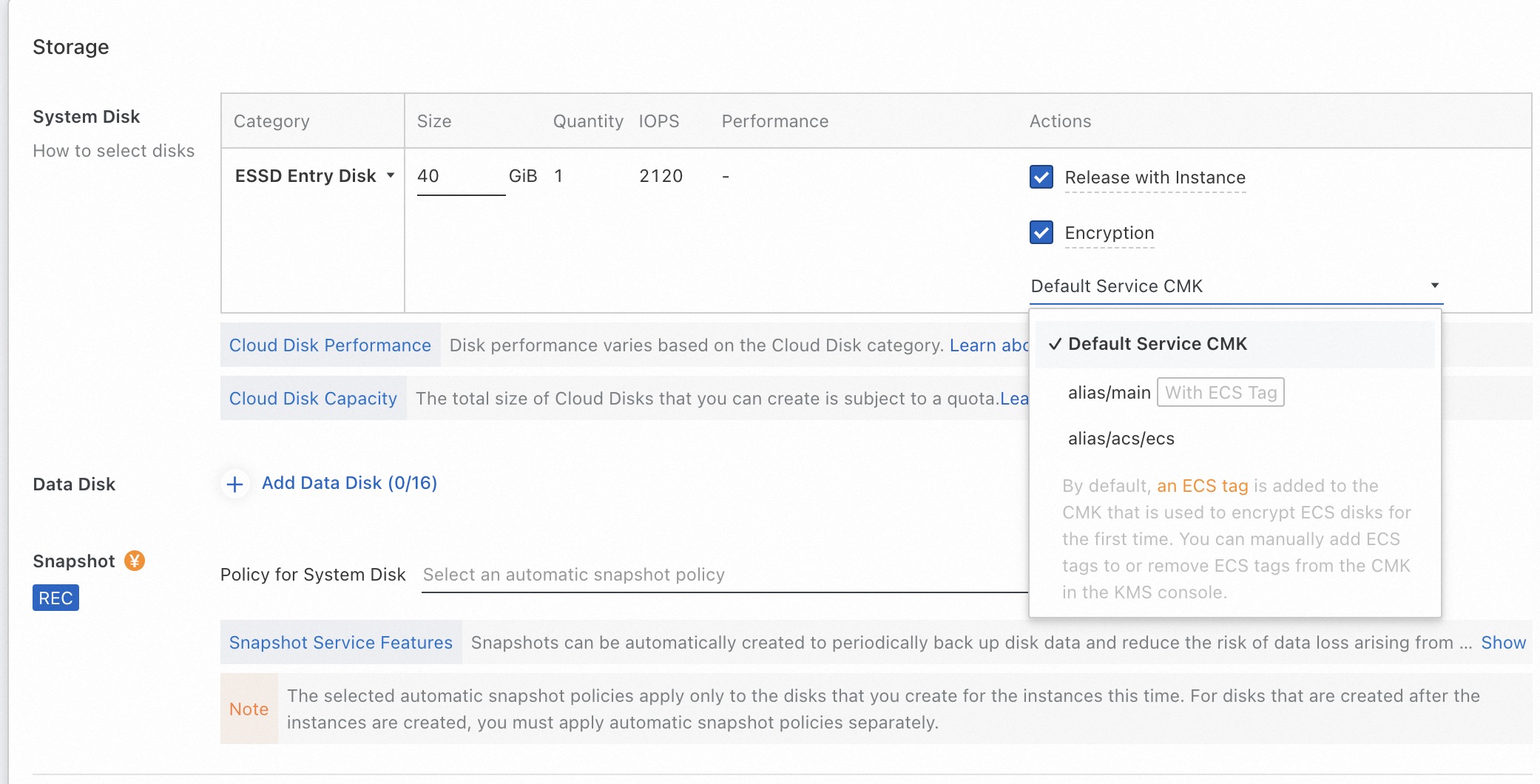
Step 3: Use KMS to encrypt data in ECS for ciphertext transmission
When data is transferred to ECS, ECS calls the API operations of KMS to encrypt and decrypt the data. You do not need to manually process the data. In most cases, Alibaba Cloud services use envelope encryption to encrypt data. For more information, see Use envelope encryption.
Application-layer cryptographic solutions
Keys can be used to build application-layer cryptographic solutions and provide API operations for client-side data encryption and decryption, and signing and verification. Key types that support this feature are software-protected keys and hardware-protected keys.
Prerequisites
A KMS instance of the hardware key management type or the software key management type is purchased and enabled. For more information, see Purchase and enable a KMS instance.
Example
In this example, a key of a KMS instance of the software key management type is used to encrypt (AdvanceEncrypt) and decrypt (AdvanceDecrypt) application-layer data. For more information, see Use a key to encrypt and decrypt data.
Step 1: Create a software-protected key
Log on to the KMS console. In the top navigation bar, select a region. In the left-side navigation pane, choose .
On the Keys tab, click Create Key and complete the configurations as prompted.
KMS Instance: Select the purchased KMS instance
Key Type: Select Symmetric Key.
Key Specifications: Select Aliyun_AES_256.
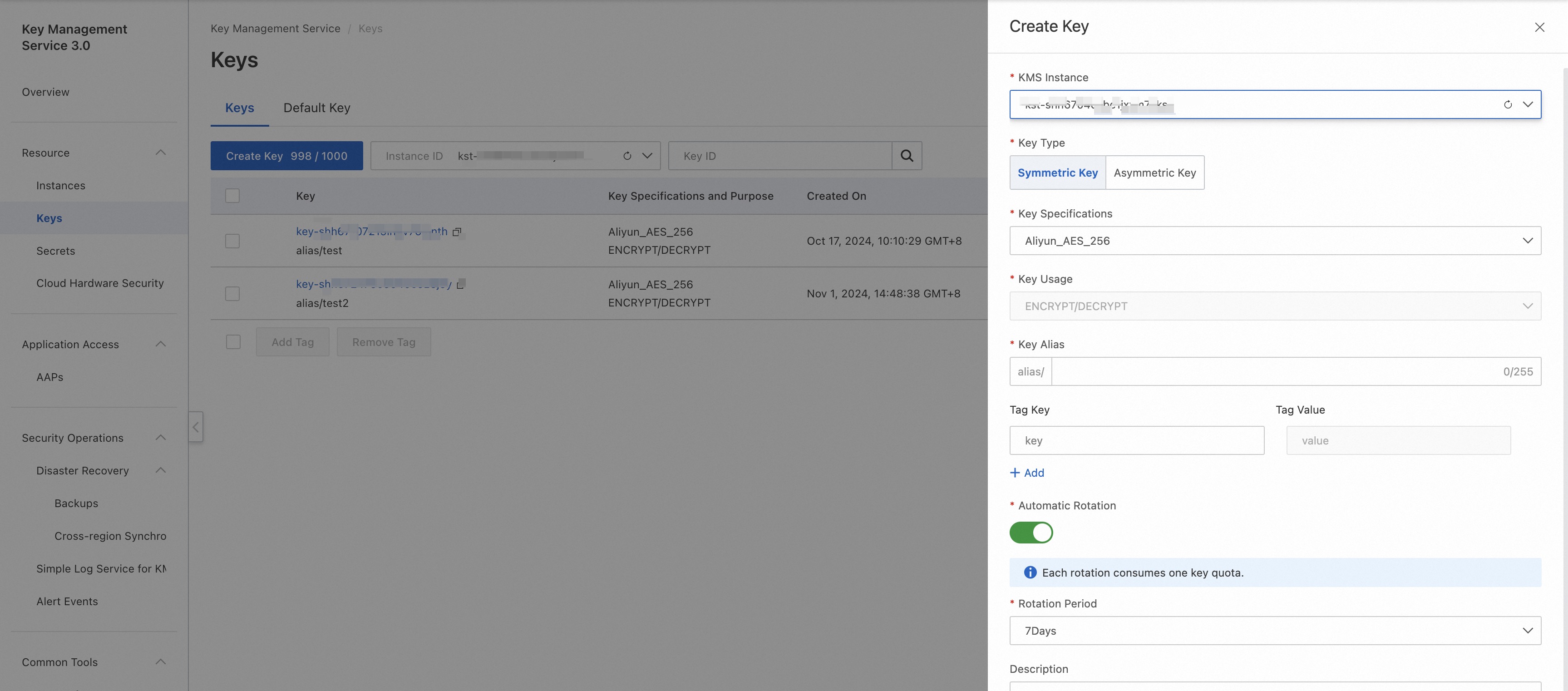
Step 2: Install dependencies
Preparations
Environment requirements
Java Development Kit (JDK) V8 or later is downloaded and installed.
Java version
Run the java -version
command on the terminal to check the JDK version.
Install an SDK
Add Maven dependencies to your project. Then, a Java package is automatically downloaded from the Maven repository.
Visit alibabacloud-dkms-gcs-java-sdk to view more installation information and the source code.
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>alibabacloud-dkms-gcs-sdk</artifactId>
<version>xx.xx.xx</version>
</dependency>
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>tea</artifactId>
<version>[1.2.3)</version>
</dependency>
Step 3: Create a credential
Log on to the KMS console. In the top navigation bar, select a region. In the left-side navigation pane, choose .
Create an application access point (AAP)
On the Application Access tab, click Create AAP. In the Create AAP panel, configure the parameters.
Parameter | Description |
Mode | Select Quick Creation. |
Scope (KMS Instance) | Select the KMS instance that you want to access. |
Application Access Point Name | Enter the name of the AAP. |
Authentication Method | The value is fixed to ClientKey. |
Default Permission Policy | The value is fixed to key/* secret/* . Your application can access all keys and secrets in the specified KMS instance. |
Click OK. The browser automatically downloads the client key that is created.
The client key contains Application Access Secret(ClientKeyContent) and Password. By default, Application Access Secret(ClientKeyContent) is saved in a file whose name is in the clientKey_****.json
format. By default, Password is saved in a file whose name is in the clientKey_****_Password.txt
format.
Step 4: Download the certificate authority (CA) certificate of the KMS instance
Log on to the KMS console. In the top navigation bar, select a region. In the left-side navigation pane, choose .
Click Download below Instance CA Certificate.
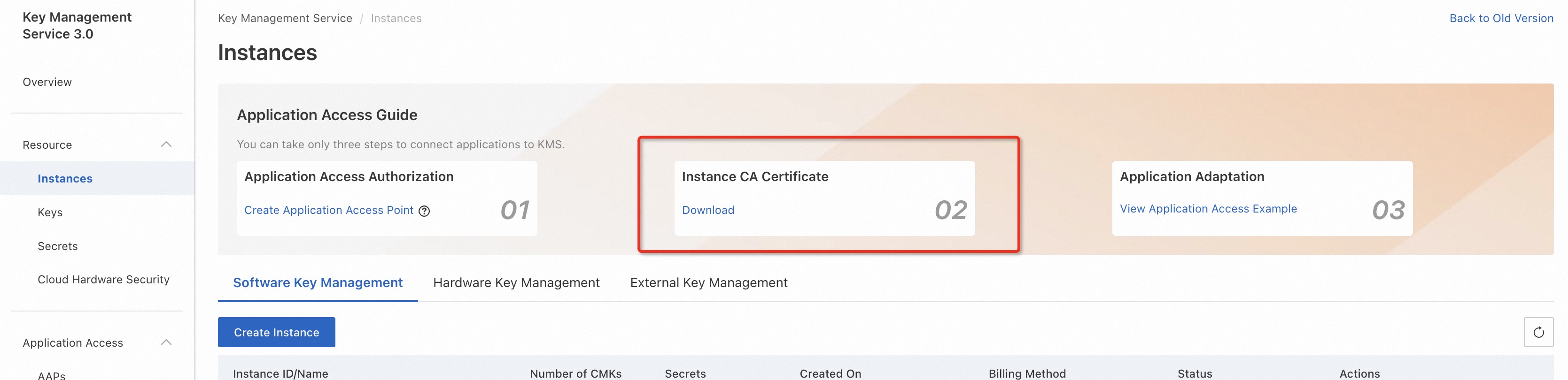
Step 5: Call API operations
Initialize KMS Instance SDK
public static void initClient() throws Exception {
Config config = new Config();
config.setProtocol("https");
config.setEndpoint("Instance ID.cryptoservice.KMS.aliyuncs.com"); // The endpoint of the KMS instance.
config.setClientKeyFile("<YOUR-CLIENT-KEY-FILE>"); // The client key file address.
// config.setClientKeyContent("<YOUR-CLIENT-KEY-CONTENT>"); // The content of the client key file. You can configure the client key file address or the content of the client key file.
config.setPassword("<YOUR-PASSWORD>"); // The password for the client key.
config.setCaFilePath("<path/to/yourCaCert>");// The CA certificate of the KMS instance. You can specify the path to the CA certificate.
// config.setCa("<your-ca-certificate-content"); // The content of the CA certificate. You can configure the path to the CA certificate or the content of the CA certificate.
client = new Client(config);
}
Call the AdvanceEncrypt operation to encrypt data.
/**
* By default, KMS uses the Galois/Counter Mode (GCM) mode.
* @param keyId You can set the keyId parameter to the key ID or the alias of a key.
* @param plaintext The data that you want to encrypt.
* @param aad You can specify the aad parameter for the GCM mode based on your business requirements. You can set the parameter to null or leave the parameter empty.
* @return The ciphertext context of the AdvanceEncrypt operation, which includes the ciphertext (ciphertextBlob) and the related parameter ciphertext (aad). In this example, AdvanceEncryptContext is used.
*/
private static AdvanceEncryptContext advanceEncryptSample(String keyId, byte[] plaintext, byte[] aad) {
AdvanceEncryptRequest request = new AdvanceEncryptRequest();
request.setKeyId(keyId);
request.setPlaintext(plaintext);
request.setAad(aad);
try {
AdvanceEncryptResponse response = client.advanceEncrypt(request);
return new AdvanceEncryptContext(response.getCiphertextBlob(), aad);
} catch (TeaException e) {
System.out.printf("code: %s%n", e.getCode());
System.out.printf("message: %s%n", e.getMessage());
System.out.printf("requestId: %s%n", e.getData().get("requestId"));
e.printStackTrace();
throw new RuntimeException(e);
} catch (Exception e) {
System.out.printf("advance encrypt err: %s%n", e.getMessage());
e.printStackTrace();
throw new RuntimeException(e);
}
}
Call the AdvanceDecrypt operation to decrypt data.
/**
* In this example, AdvanceDecrypt is used.
*
* @param advanceEncryptContext The ciphertext context of the AdvanceEncrypt operation, which includes the ciphertext (ciphertextBlob) and related parameter ciphertext (aad). In this example, AdvanceEncryptContext is used.
* @return The plaintext that is obtained after decryption.
*/
private static byte[] advanceDecryptSample(final AdvanceEncryptContext advanceEncryptContext) {
// Build an advanced decryption request object.
AdvanceDecryptRequest request = new AdvanceDecryptRequest();
request.setCiphertextBlob(advanceEncryptContext.getCiphertextBlob());
request.setAad(advanceEncryptContext.getAad());
try {
AdvanceDecryptResponse response = client.advanceDecrypt(request);
System.out.printf("RequestId: %s%n", response.getRequestId());
return response.getPlaintext();
} catch (TeaException e) {
System.out.printf("code: %s%n", e.getCode());
System.out.printf("message: %s%n", e.getMessage());
System.out.printf("requestId: %s%n", e.getData().get("requestId"));
e.printStackTrace();
throw new RuntimeException(e);
} catch (Exception e) {
System.out.printf("advance decrypt err: %s%n", e.getMessage());
e.printStackTrace();
throw new RuntimeException(e);
}
}
For more information about complete code sample, see AdvanceEncryptAdvanceDecryptSample.java.