If you use the web tracking SDK for JavaScript to upload logs, you must enable the web tracking feature for the Logstore. This may generate dirty data records. Alibaba Cloud Security Token Service (STS) allows you to manage temporary credentials to your Alibaba Cloud resources. You can use STS to obtain temporary credentials (STS tokens) with custom validity periods and access permissions. You can use the STS plug-in of web tracking SDK for JavaScript to upload logs. In this case, you do not need to enable the web tracking feature for the Logstore.
Background information

If you do not use STS for temporary authorization, you must enable the web tracking feature for the Logstore. Web tracking SDK for JavaScript uploads logs collected from browsers to the Logstore of Simple Log Service, which may generate dirty data. The following items describe the process of using the STS plug-in of web tracking SDK for JavaScript to upload logs:
A browser requests a temporary identity credential (STS token) from a business server.
The service server requests an STS token from the STS service.
An AccessKey pair of the Resource Access Management (RAM) user is configured in the server and the RAM user is attached the AliyunSTSAssumeRoleAccess policy.
The server uses the AccessKey pair of the RAM user to call the AssumeRole operation of STS to obtain the STS token of the RAM role. You can use the Policy
parameter of AssumeRole to restrict the permissions of different STS tokens based on the user or device.
STS returns an STS token to the business server.
The service server returns an STS token to the browser.
The browser uses the obtained STS token to assume the RAM role and uploads logs collected from the browser to the Logstore.
Simple Log Service returns a response to the browser after the log is uploaded.
Step 1: Install and configure the SDK
Install Node.js.
Run the following command on the business server to install dependencies.
npm install --save @aliyun-sls/web-track-browser
npm install --save @aliyun-sls/web-sts-plugin
Add the following code to your program to configure the SDK.
import SlsTracker from '@aliyun-sls/web-track-browser'
import createStsPlugin from '@aliyun-sls/web-sts-plugin'
const opts = {
host: '${host}',
project: '${project}',
logstore: '${logstore}',
time: 10,
count: 10,
topic: 'topic',
source: 'source',
tags: {
tags: 'tags',
},
}
const stsOpt = {
accessKeyId: '',
accessKeySecret: '',
securityToken: '',
refreshSTSToken: () =>
new Promise((resolve, reject) => {
const xhr = new window.XMLHttpRequest()
xhr.open('GET', 'localhost:7000/test/sts', true)
xhr.send()
xhr.onreadystatechange = () => {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
let credential = JSON.parse(xhr.response)
stsOpt.accessKeyId = credential.AccessKeyId
stsOpt.accessKeySecret = credential.AccessKeySecret
stsOpt.securityToken = credential.SecurityToken
resolve()
} else {
reject('Wrong status code.')
}
}
}
}),
}
const tracker = new SlsTracker(opts)
const stsPlugin = createStsPlugin(stsOpt)
tracker.useStsPlugin(stsPlugin)
tracker.send({
eventType:'view_product',
productName: 'Tablet',
price: 500
})
The description of web tracking parameters is as follows:
Parameter | Required | Description |
Parameter | Required | Description |
host | Yes | The endpoint of the region where Simple Log Service resides. In this example, the Simple Log Service endpoint for the China (Hangzhou) region is used. Replace the parameter value with the actual endpoint. For more information, see Endpoints. |
project | Yes | The name of the project. |
logstore | Yes | The name of the Logstore. |
time | No | The interval at which logs are sent. Default value: 10. Unit: seconds. |
count | No | The maximum number of logs that can be sent in each request. Default value: 10. |
topic | No | The topic of logs. You can specify a custom value for this parameter to facilitate identification. |
source | No | The source of logs. You can specify a custom value for this parameter to facilitate identification. |
tags | No | The tag information about logs. You can specify a custom tag to identify logs. |
The description of STS parameters is as follows:
Parameter | Required | Description |
Parameter | Required | Description |
accessKeyId accessKeySecret securityToken
| Yes | The business server uses the AccessKey pair of the RAM user to call the AssumeRole operation. The response parameters include AccessKeySecret , AccessKeyId , and SecurityToken . In the input parameters of the AssumeRole operation, DurationSeconds specifies the validity period of the token and Policy specifies the permission scope of the token.
|
refreshSTSToken | Yes | Your STS token request function, which is used to periodically obtain STS tokens to update the preceding fields. You can use a Promise/async function. |
refreshSTSTokenInterval | No | The interval at which tokens are refreshed, in millisecond. Default value: 300,000, which indicates 5 minutes. |
stsTokenFreshTime | No | The latest token acquisition time. You do not need to specify this parameter. |
Step 2: Upload logs
When you upload a single log, the log is uploaded as a separate object
. When you upload multiple logs, the logs are uploaded as an array
that contains multiple objects
.
Upload a single log. The type is object
. Example:
tracker.send({
eventType:'view_product',
productName: 'Tablet',
price: 500
})
Immediately upload a single log. The time and count parameters do not take effect. The type is object
. Example:
tracker.sendImmediate({
eventType:'view_product',
productName: 'Tablet',
price: 500
})
Upload multiple logs at a time. The type is array
. Example:
tracker.sendBatchLogs([
{
eventType: 'view_product',
productName: 'Tablet',
price: 500
},
{
eventType: 'view_product',
productName: 'Laptop',
price: 1200
}
])
Immediately upload multiple logs at a time. The time and count parameters do not take effect. The type is array
. Example:
tracker.sendBatchLogsImmediate([
{
eventType:'view_product',
productName: 'Tablet',
price: 500
},
{
eventType:'view_product',
productName: 'Laptop',
price: 1200
}
])
Step 3: View the upload result
After logs are uploaded to a Logstore, you must create indexes to query and analyze the logs. For more information, see Create indexes.
Quick view
If no indexes are created, you can click Consumption Preview to quickly view logs. Then, you can query and analyze the logs. For more information, see Query and analyze logs.
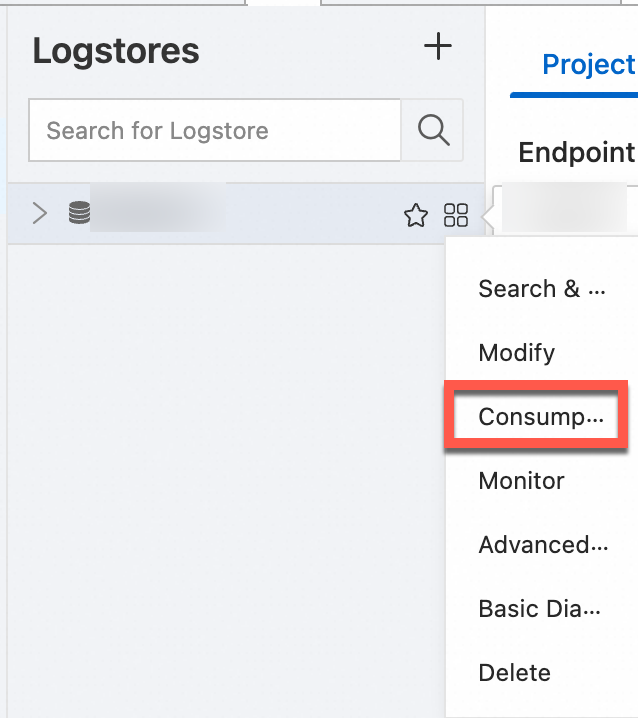
Query and analyze logs
You can call the CreateIndex operation to create full-text indexes or field indexes. If you want to use the SELECT statement, you must create field indexes.
You can call the GetLogsV2 operation to query logs in a Logstore. The returned result is an array of logs. Each element in the array is a log.
FAQ
The SDK starts without errors, but when sending logs, it reports the TypeError: Cannot read properties of undefined (reading 'sigBytes')
. What do I do if this issue occurs?
Possible cause: Issues with CryptoJS encryption. Due to the difficulty in modifying the third-party package, we recommend that you use web tracking for unauthenticated writes. For more information, see Use the web tracking feature to collect logs.