This topic provides sample code that shows how to request a JavaScript file and return the file to the client.
Sample code
/**
* The sample code shows how to request the file https://demo.aliyundoc.com/alipay/qrcode/1.0.3/qrcode.js and return the file to the client.
* Replace someHost and url with your host and URL addresses during the test.
*/
const someHost = "https://demo.aliyundoc.com/alipay/qrcode/1.0.3/"
const url = someHost + "qrcode.js"
/**
* Use gatherResponse to obtain the response body and return it to the client as a string.
* Use await gatherResponse(..) in an asynchronous function to ensure that the whole response body is obtained.
*/
async function gatherResponse(response) {
const headers = response.headers
const contentType = headers.get("content-type") || ""
if (contentType.includes("application/json")) {
return JSON.stringify(await response.json())
} else if (contentType.includes("application/text")) {
return response.text()
} else if (contentType.includes("text/html;charset=UTF-8")) {
return response.text()
} else {
return response.blob()
}
}
async function handleRequest() {
const init = {
headers: {
"content-type": "application/json;charset=UTF-8",
},
}
const response = await fetch(url, init)
const results = await gatherResponse(response)
return new Response(results, init)
}
addEventListener("fetch", event => {
return event.respondWith(handleRequest())
})
Result
A request to fetch the file https://demo.aliyundoc.com/alipay/qrcode/1.0.3/qrcode.js
is sent in EdgeRoutine and the file is returned to the client.
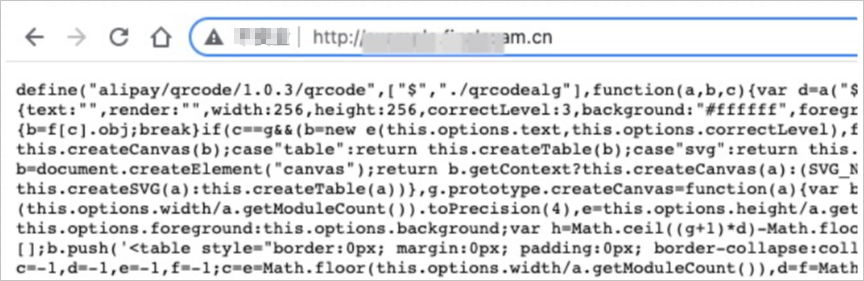